python cv2 实现 读取图片,在图片指定范围内随机打点,并批量保存被打点图片
时间: 2024-03-27 19:40:26 浏览: 5
您可以按照以下步骤实现您的需求:
1. 引入必要的库和函数:
```python
import cv2
import numpy as np
import os
```
2. 定义读取图片函数:
```python
def read_image(image_path):
image = cv2.imread(image_path)
return image
```
3. 定义打点函数:
```python
def draw_random_points(image, n_points, x_range, y_range):
for i in range(n_points):
x = np.random.randint(x_range[0], x_range[1])
y = np.random.randint(y_range[0], y_range[1])
cv2.circle(image, (x, y), 1, (0, 0, 255), -1)
return image
```
其中,参数 `n_points` 表示要在图片中打多少个点,`x_range` 和 `y_range` 分别表示点的横坐标和纵坐标的范围。
4. 定义批量保存函数:
```python
def save_images(images, output_dir):
if not os.path.exists(output_dir):
os.makedirs(output_dir)
for i, image in enumerate(images):
output_path = os.path.join(output_dir, f"{i+1}.jpg")
cv2.imwrite(output_path, image)
```
其中,参数 `images` 表示要保存的图片列表,`output_dir` 表示保存的目录。
5. 主函数中读取图片、打点并保存:
```python
if __name__ == '__main__':
image_path = "test.jpg"
output_dir = "output"
n_points = 100
x_range = (100, 300)
y_range = (200, 400)
image = read_image(image_path)
images = []
for i in range(10):
new_image = draw_random_points(image.copy(), n_points, x_range, y_range)
images.append(new_image)
save_images(images, output_dir)
```
其中,`image_path` 表示要读取的图片路径,`output_dir` 表示保存的目录,`n_points` 表示要在每张图片中打多少个点,`x_range` 和 `y_range` 分别表示点的横坐标和纵坐标的范围。上述代码中,我们将在一张图片中打 100 个点,重复 10 次并保存。您可以根据自己的需求进行修改。
相关推荐
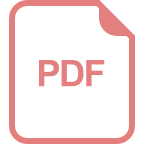














