多字下 const char* str = "E:\\0000\\夜鸦\\版本完整包\\巴尔\\BE.dll"; 修改为获取当前目录下BE.dll的目录
时间: 2024-03-15 11:39:59 浏览: 121
要获取当前目录下BE.dll的目录,可以使用以下方法:
1. 使用C++的文件操作函数:
```cpp
#include <iostream>
#include <filesystem>
int main() {
std::filesystem::path filePath = std::filesystem::current_path() / "BE.dll";
std::string directory = filePath.parent_path().string();
std::cout << directory << std::endl;
return 0;
}
```
2. 使用C的文件操作函数:
```cpp
#include <stdio.h>
#include <stdlib.h>
#include <direct.h>
int main() {
char buffer[FILENAME_MAX];
_getcwd(buffer, sizeof(buffer));
strcat(buffer, "\\BE.dll");
printf("%s\n", buffer);
return 0;
}
```
这两种方法都可以获取当前目录下BE.dll的目录。第一种方法使用了C++17中的std::filesystem库,需要编译器支持。第二种方法使用了C标准库中的_getcwd函数,适用于C和C++。
相关问题
C++多字节判断此文件是否存在 E:\0000\夜鸦\版本完整包\巴尔\AccountConfig\1Qqtzh1@pccprep.online\Config.ini
在C++中,可以使用多字节字符集来处理包含非ASCII字符的文件路径。要判断文件是否存在,可以使用以下步骤:
1. 使用`MultiByteToWideChar`函数将多字节字符转换为宽字符。
2. 使用`_waccess`函数检查文件是否存在。
下面是一个示例代码:
```cpp
#include <iostream>
#include <Windows.h>
bool FileExists(const char* filePath)
{
// 将多字节字符转换为宽字符
int wideCharLen = MultiByteToWideChar(CP_UTF8, 0, filePath, -1, nullptr, 0);
wchar_t* wideFilePath = new wchar_t[wideCharLen];
MultiByteToWideChar(CP_UTF8, 0, filePath, -1, wideFilePath, wideCharLen);
// 检查文件是否存在
bool exists = (_waccess(wideFilePath,0) != -1);
delete[] wideFilePath;
return exists;
}
int main()
{
const char* filePath = "E:\\0000\\夜鸦\\版本完整包\\巴尔\\AccountConfig\\1Qqtzh1@pccprep.online\\Config.ini";
if (FileExists(filePath))
{
std::cout << "文件存在" << std::endl;
}
else
{
std::cout << "文件不存在" << std::endl;
}
return 0;
}
```
阅读全文
相关推荐
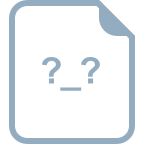
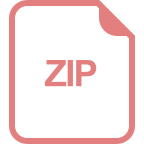
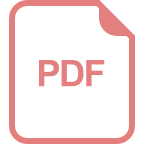
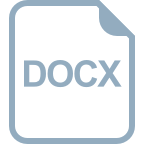
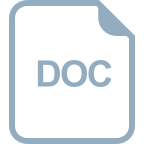
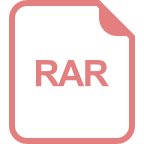
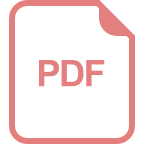
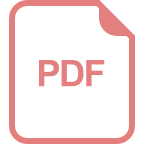
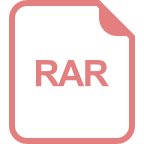
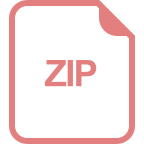
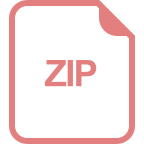
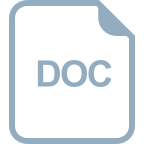
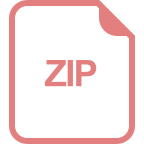
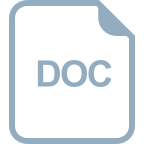
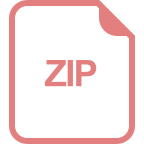
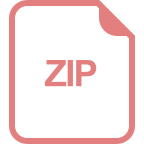