python 实现otsu阈值法
时间: 2024-12-23 13:13:07 浏览: 7
在 Python 中,你可以使用 `skimage` 库中的 `skimage.filters.threshold_otsu()` 函数来应用 Otsu 阈值分割算法。Otsu's method 是一种二值化图像的方法,它自动寻找最佳阈值,使得图像中最暗区域和最亮区域之间的方差最小。
以下是一个简单的示例,演示如何使用 skimage 库实现 Otsu 阈值法:
```python
from skimage import filters, img_as_ubyte
import numpy as np
def apply_otsu_threshold(image_path):
# 读取图像数据
image = cv2.imread(image_path, cv2.IMREAD_GRAYSCALE) # 注意这里假设是灰度图像
if image.ndim == 3: # 如果是彩色图像,先转为灰度
image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 应用 Otsu 阈值
otsu_threshold = filters.threshold_otsu(image)
# 使用阈值对图像进行二值化
binary_image = np.where(image > otsu_threshold, 255, 0).astype(np.uint8) # 通常设置背景为0,前景为255
return binary_image
# 示例
binary_image = apply_otsu_threshold("test.png")
cv2.imshow("Otsu Threshold Binary Image", binary_image)
cv2.waitKey(0) # 等待用户按键,按任意键关闭窗口
cv2.destroyAllWindows()
```
在这个例子中,`img_as_ubyte()` 是为了确保输出结果为无符号8位整型数组,因为 Otsu 方法返回的是浮点类型的阈值。
阅读全文
相关推荐
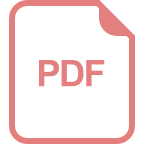
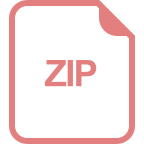



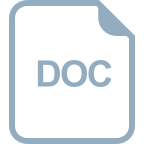
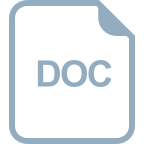
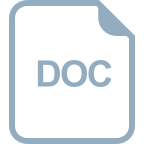









