C语言设计一个算法来判断一个字符串是否为回文。假设字符串从标准输入设备一次读入一个字符,算法的输出结果为true或者false。可以用一些字符串测试输出结果,如: "abcdeabcde", "madamimadam" 等。
时间: 2024-12-19 08:25:35 浏览: 5
在C语言中,判断一个字符串是否为回文可以使用双指针法,即一个指向字符串开头,另一个指向结尾,逐个比较字符直到两个指针相遇或交叉。如果在整个过程中所有字符都匹配,则该字符串是回文。以下是详细的算法:
```c
#include <stdio.h>
#include <stdbool.h>
#include <string.h>
bool isPalindrome(char str[]) {
int start = 0;
int end = strlen(str) - 1; // 注意字符串结束索引
// 只要开始位置小于等于结束位置,就继续比较
while (start < end) {
// 如果字符不相等,返回false
if (str[start] != str[end]) {
return false;
}
// 移动指针
start++;
end--;
}
// 所有字符都匹配,返回true
return true;
}
int main() {
char test1[] = "abcdeabcde";
char test2[] = "madamimadam";
if (isPalindrome(test1)) {
printf("%s is a palindrome.\n", test1);
} else {
printf("%s is not a palindrome.\n", test1);
}
if (isPalindrome(test2)) {
printf("%s is a palindrome.\n", test2);
} else {
printf("%s is not a palindrome.\n", test2);
}
return 0;
}
```
在这个程序中,`isPalindrome()`函数通过遍历字符串的一半并与另一半进行比较来确定是否为回文。如果找到不相等的字符,它立即返回`false`;如果没有找到不相等的字符,表示整个字符串是回文,所以返回`true`。
阅读全文
相关推荐
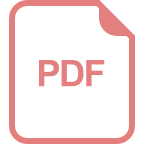
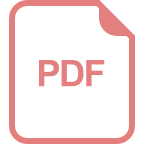
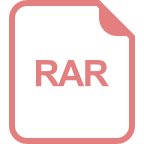



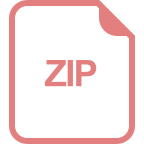











