if order_response[0]['data'][0]['state'] == 'filled': TypeError: 'NoneType' object is not subscriptable
时间: 2023-10-14 14:04:52 浏览: 137
这个错误通常发生在尝试对空对象进行索引时。具体来说,在这个代码行中, `order_response` 可能是空值,或者 `order_response[0]['data']` 可能是空值。
要解决这个问题,可以在使用 `order_response` 之前先检查它是否为空。例如:
```python
if order_response is not None and order_response[0]['data'] is not None and order_response[0]['data'][0]['state'] == 'filled':
# 进行相应的操作
else:
# 处理空对象的情况
```
这样做可以避免对空对象进行索引,从而避免 TypeError 异常。
相关问题
解释这段代码:# 决策树 dt = DecisionTreeClassifier(max_depth=5, random_state=0) dt.fit(X_train, y_train) y_pred_dt = dt.predict(X_test) print('决策树准确率:', metrics.accuracy_score(y_test, y_pred_dt)) # 决策树可视化 dot_data = export_graphviz(dt, out_file=None, feature_names=X_train.columns, class_names=['Dead', 'Survived'], filled=True, rounded=True, special_characters=True) graph = graphviz.Source(dot_data) graph.render('titanic_decision_tree') # 剪枝 dt_pruned = DecisionTreeClassifier(max_depth=5, ccp_alpha=0.01, random_state=0) dt_pruned.fit(X_train, y_train) y_pred_pruned = dt_pruned.predict(X_test) print('剪枝决策树准确率:', metrics.accuracy_score(y_test, y_pred_pruned)) # 随机森林 rf = RandomForestClassifier(n_estimators=100, max_depth=5, random_state=0) rf.fit(X_train, y_train) y_pred_rf = rf.predict(X_test) print('随机森林准确率:', metrics.accuracy_score(y_test, y_pred_rf))
这段代码是用于构建和比较不同决策树和随机森林模型的分类准确率。首先,使用DecisionTreeClassifier函数构建一个决策树模型,设置最大深度为5,随机种子为0,并使用X_train和y_train训练模型,使用X_test预测结果并计算准确率。然后,使用export_graphviz函数将决策树可视化,设置特征名称为X_train的列名,类别名称为Dead和Survived,并将结果图形保存为titanic_decision_tree。接着,使用DecisionTreeClassifier函数构建一个剪枝决策树模型,除了最大深度为5外,还设置了ccp_alpha参数为0.01,并使用X_train和y_train训练模型,使用X_test预测结果并计算准确率。最后,使用RandomForestClassifier函数构建一个随机森林模型,设置树的数量为100,最大深度为5,随机种子为0,并使用X_train和y_train训练模型,使用X_test预测结果并计算准确率。
from sklearn import tree tree.export_graphviz(classifier,out_file="tree.dot" ) import pydotplus from IPython.display import Image dot_data=tree.export_graphviz(classifier, out_file=None,feature_names=irisdata.feature_names,filled=True, rounded=True,special_characters=True) graph = pydotplus.graph_from_dot_data(dot_data) 帮我画出决策树
很高兴为您提供帮助!以下是绘制决策树的代码:
```python
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
from sklearn import tree
import pydotplus
from IPython.display import Image
# 加载数据集
iris = load_iris()
X = iris.data
y = iris.target
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, random_state=0)
# 创建决策树模型
classifier = DecisionTreeClassifier(random_state=0)
classifier.fit(X_train, y_train)
# 导出决策树图
dot_data = tree.export_graphviz(classifier, out_file=None,
feature_names=iris.feature_names,
class_names=iris.target_names,
filled=True, rounded=True,
special_characters=True)
graph = pydotplus.graph_from_dot_data(dot_data)
Image(graph.create_png())
```
运行以上代码,即可绘制出决策树图。您可以根据需要将图形保存为PNG或PDF文件。
阅读全文
相关推荐
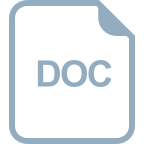
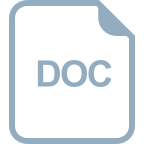
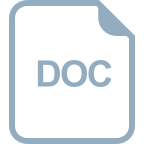














