PCL汉明距离特征点匹配代码
时间: 2023-07-10 12:12:56 浏览: 160
下面是使用PCL库实现汉明距离特征点匹配的代码示例:
```cpp
#include <pcl/point_types.h>
#include <pcl/features/feature.h>
#include <pcl/features/fpfh.h>
#include <pcl/features/shot.h>
#include <pcl/registration/correspondence_estimation.h>
#include <pcl/registration/correspondence_rejection_one_to_one.h>
#include <pcl/registration/transformation_estimation_svd.h>
// 定义点云类型
typedef pcl::PointXYZ PointT;
typedef pcl::PointCloud<PointT> PointCloud;
int main()
{
// 读取点云A和点云B
PointCloud::Ptr cloudA(new PointCloud);
pcl::io::loadPCDFile("cloudA.pcd", *cloudA);
PointCloud::Ptr cloudB(new PointCloud);
pcl::io::loadPCDFile("cloudB.pcd", *cloudB);
// 初始化特征估计器和匹配器
pcl::SHOTEstimation<PointT, pcl::Normal, pcl::SHOT352> shotEstimator;
pcl::CorrespondenceEstimation<pcl::SHOT352, pcl::SHOT352> correspondenceEstimator;
pcl::CorrespondenceRejectorOneToOne rejector;
pcl::TransformationEstimationSVD<PointT, PointT> transformationEstimator;
// 计算点云A和点云B的SHOT特征
pcl::PointCloud<pcl::Normal>::Ptr normalsA(new pcl::PointCloud<pcl::Normal>);
pcl::PointCloud<pcl::Normal>::Ptr normalsB(new pcl::PointCloud<pcl::Normal>);
pcl::NormalEstimation<PointT, pcl::Normal> normalEstimator;
normalEstimator.setInputCloud(cloudA);
normalEstimator.setRadiusSearch(0.02);
normalEstimator.compute(*normalsA);
shotEstimator.setInputCloud(cloudA);
shotEstimator.setInputNormals(normalsA);
pcl::PointCloud<pcl::SHOT352>::Ptr descriptorsA(new pcl::PointCloud<pcl::SHOT352>);
shotEstimator.compute(*descriptorsA);
normalEstimator.setInputCloud(cloudB);
normalEstimator.compute(*normalsB);
shotEstimator.setInputCloud(cloudB);
shotEstimator.setInputNormals(normalsB);
pcl::PointCloud<pcl::SHOT352>::Ptr descriptorsB(new pcl::PointCloud<pcl::SHOT352>);
shotEstimator.compute(*descriptorsB);
// 计算点云A和点云B之间的匹配
correspondenceEstimator.setInputSource(descriptorsA);
correspondenceEstimator.setInputTarget(descriptorsB);
pcl::CorrespondencesPtr correspondences(new pcl::Correspondences);
correspondenceEstimator.determineCorrespondences(*correspondences);
// 去掉不合理的匹配
rejector.setInputCorrespondences(correspondences);
pcl::CorrespondencesPtr inliers(new pcl::Correspondences);
rejector.getCorrespondences(*inliers);
// 估计刚体变换
Eigen::Matrix4f transformation;
transformationEstimator.estimateRigidTransformation(*cloudA, *cloudB, *inliers, transformation);
return 0;
}
```
上述代码中使用了PCL库中的SHOT特征估计器、匹配器和刚体变换估计器等模块,实现了点云的特征点匹配。需要注意的是,由于SHOT特征是基于点法线的,因此在计算SHOT特征之前需要先计算点云的法线。在实际应用中,可能需要根据具体的应用场景选择不同的特征估计器和匹配器,并结合其他优化方法来提高匹配的准确性。
阅读全文
相关推荐
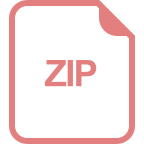
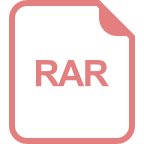
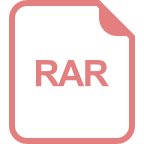










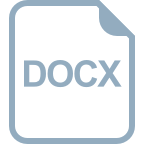