int indexCity(std::string cityName, int number = 0) { for (int i = 0; i < MAXCITY; i++) { if (data->city[i].name == cityName || data->city[i].number == number) { return i; } } return -1; }
时间: 2024-03-28 18:41:03 浏览: 17
这段代码看起来像是一个函数定义,函数名为 indexCity,接受两个参数:一个字符串类型的 cityName 和一个整数类型的 number,其中 number 参数有默认值为 0。函数中使用了一个 for 循环来遍历一个名为 data 的对象的 city 数组,如果数组中的某个元素的 name 属性等于 cityName 或者 number 属性等于 number 参数,则返回该元素的索引值 i。如果循环结束后仍没有找到符合条件的元素,则返回 -1。根据代码的上下文,MAXCITY 可能是一个预定义的常量,表示数组 city 的最大长度。
相关问题
#include <iostream> #include <fstream> #include <vector> #include <algorithm> using namespace std; // 城市数据的结构体 struct CityData { int cityIndex; // 城市序号 string cityName; // 城市名称 double gdp; // 城市GDP总量 int population; // 城市常驻人口数 }; // 用于比较城市GDP的排序谓词 bool compareByGDP(const CityData& city1, const CityData& city2) { return city1.gdp > city2.gdp; // 按照GDP从大到小排序 } int main() { vector<CityData> cityDataList; // 存储城市数据的向量 ifstream inputFile("gdp_data.txt"); // 打开数据文件 if (!inputFile) { cout << "无法打开文件 gdp_data.txt" << endl; return 1; } int cityIndex; string cityName; double gdp; int population; // 读取文件中的数据并存储到结构体中 while (inputFile >> cityIndex >> cityName >> gdp >> population) { CityData cityData; cityData.cityIndex = cityIndex; cityData.cityName = cityName; cityData.gdp = gdp; cityData.population = population; cityDataList.push_back(cityData); } inputFile.close(); // 关闭文件 // 统计GDP最高的4个城市 cout << "GDP总量最高的4个城市:" << endl; for (int i = 0; i < 4; i++) { cout << cityDataList[i].cityName << ": " << cityDataList[i].gdp << "亿元" << endl; } cout << endl; // 统计GDP最低的4个城市 cout << "GDP总量最低的4个城市:" << endl; int size = cityDataList.size(); for (int i = size - 4; i < size; i++) { cout << cityDataList[i].cityName << ": " << cityDataList[i].gdp << "亿元" << endl; } cout << endl; // 计算8个城市的GDP和占全省GDP总量的比例 double totalGDP = 0.0; for (const auto& cityData : cityDataList) { totalGDP += cityData.gdp; } double selectedCitiesGDP = 0.0; for (int i = 0; i < 8; i++) { selectedCitiesGDP += cityDataList[i].gdp; } double percentage = (selectedCitiesGDP / totalGDP) * 100; cout << "8个城市的GDP总量占全省GDP总量的比例:" << percentage << "%" << endl; return 0; }解释这段代码
这段代码是一个 C++ 程序,用于读取一个文本文件中的城市数据,包括城市序号、城市名称、GDP总量和常驻人口数,并统计分析这些数据。程序首先定义了一个结构体 `CityData`,用于存储城市数据。然后定义了一个排序谓词 `compareByGDP`,用于按照城市的 GDP 从大到小排序。程序使用了 STL 中的向量 `vector` 来存储城市数据,读取文件中的数据后将其存储到向量中。程序输出了 GDP 总量最高的 4 个城市和 GDP 总量最低的 4 个城市的名称和 GDP 值,并计算了 8 个城市的 GDP 总量占全省 GDP 总量的比例。
int i=1; while (rs.next()) { System.out.println(i++); String json = "{" + "\"cityName\":" + "\"" + rs.getString(1) + "\"" + "," + "\"value\":" + "\"" + rs.getInt(2) + "\"" + "}"; result = result + json + ","; } result = "[" + result.substring(0, result.length() - 1) + "]";
这段代码看起来是Java代码。它的作用是从数据库中查询出城市名称和对应的数值,然后将它们拼接成JSON字符串的形式,最后将这些JSON字符串拼接成一个JSON数组返回。其中,使用了ResultSet对象的next()方法遍历数据库查询结果,使用了字符串拼接来构造JSON字符串,并使用了字符串截取来去掉最后一个逗号并在两端添加方括号构成JSON数组。
相关推荐
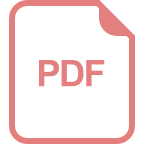
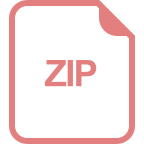
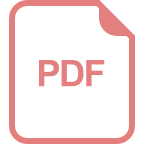













