dijkstra算法有向图python代码
时间: 2023-07-23 16:42:35 浏览: 49
下面是使用Dijkstra算法在有向图中寻找最短路径的Python代码示例:
```python
import heapq
def dijkstra(graph, start):
# 初始化距离字典
distances = {node: float('inf') for node in graph}
distances[start] = 0
# 使用堆来维护待处理的节点
heap = [(0, start)]
while heap:
# 取出当前堆中最小的距离和节点
current_distance, current_node = heapq.heappop(heap)
# 如果当前距离已经大于当前节点到起点的最短距离,则直接跳过
if current_distance > distances[current_node]:
continue
# 遍历当前节点的所有邻居节点
for neighbor, weight in graph[current_node].items():
# 计算邻居节点到起点的距离
distance = current_distance + weight
# 如果新的距离比已知的最短距离还要短,则更新距离字典和堆中的节点
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(heap, (distance, neighbor))
return distances
```
使用示例:
```python
graph = {
'A': {'B': 5, 'C': 1},
'B': {'D': 2},
'C': {'B': 3, 'D': 4},
'D': {'E': 6},
'E': {}
}
start = 'A'
print(dijkstra(graph, start))
```
输出结果为:
```
{'A': 0, 'B': 4, 'C': 1, 'D': 6, 'E': 12}
```
以上代码实现了Dijkstra算法的基本逻辑,可以在有向图中找到起点到所有节点的最短路径。需要注意的是,该代码中使用了堆来维护待处理的节点,以提高算法的效率。
相关推荐
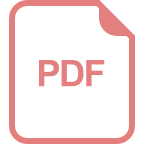













