C语言建立俩个顺序表(通过随机函数生成)
时间: 2024-10-05 17:04:10 浏览: 38
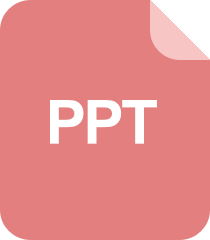
fseek函数和随机读写-C语言入门教程
在C语言中,创建两个顺序表通常涉及到结构体来存储元素以及动态内存分配。这里是一个简单的步骤说明:
1. **定义数据结构**:
首先,定义一个结构体(struct)来表示列表中的元素,比如整数:
```c
struct ListElement {
int data;
struct ListElement* next; // 指向下一个元素的指针
};
```
2. **生成随机数**:
使用`<stdlib.h>`库的`rand()`和`srand()`函数来生成随机数,例如:
```c
#include <time.h>
srand(time(0)); // 设置随机数种子
int generate_random(int min, int max) {
return (min + rand() % (max - min + 1));
}
```
3. **创建顺序表**:
创建两个顺序表并初始化它们,可以分别使用全局变量或函数局部变量:
```c
struct ListElement* list1 = NULL;
struct ListElement* list2 = NULL;
void create_list(int size, int min, int max) {
for (int i = 0; i < size; ++i) {
struct ListElement* newNode = (struct ListElement*)malloc(sizeof(struct ListElement));
newNode->data = generate_random(min, max);
newNode->next = NULL;
if (!list1) {
list1 = newNode;
} else {
list1->next = newNode;
}
}
}
// 对第二个列表做同样的操作
void create_list2(int size, int min, int max) {
// ...
}
```
4. **插入和遍历顺序表**:
对于添加元素到已存在的列表,可以按照类似的方式添加。
阅读全文
相关推荐
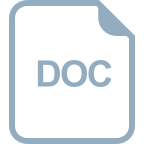
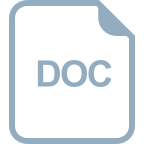


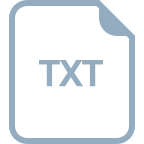
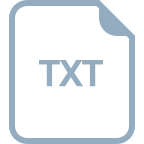
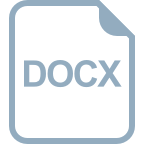
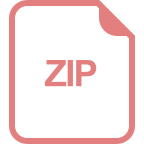
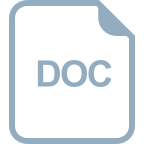
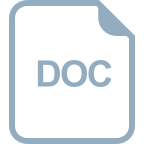
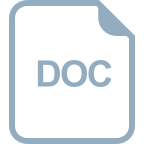
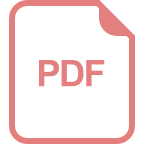
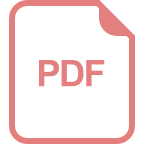


