Write a Python function to check whether a string is pangram or not. (Assume the string passed in does not have any punctuation)
时间: 2024-10-13 12:03:25 浏览: 27
当然可以,首先你需要了解什么是帕格拉姆(Pangram),它是指包含英语字母表所有26个字母的句子或短语。下面是一个简单的Python函数,用于检查给定字符串是否为帕格拉姆:
```python
def is_pangram(s):
alphabet = "abcdefghijklmnopqrstuvwxyz"
for char in alphabet:
if char not in s.lower():
return False
return True
# 测试示例
s = "The quick brown fox jumps over the lazy dog"
if is_pangram(s):
print(f"{s} is a pangram.")
else:
print(f"{s} is not a pangram.")
# 使用这个函数检查输入的字符串是否为帕格拉姆
input_string = input("Enter a string without punctuation: ")
if is_pangram(input_string):
print("The string is a pangram.")
else:
print("The string is not a pangram.")
相关问题
Implement about, which takes a list of topic words. It returns a function that can be passed to choose as the select argument. The returned function takes a paragraph and returns a boolean indicating whether that paragraph contains any of the words in topic. To make this comparison accurately, you will need to ignore case (that is, assume that uppercase and lowercase letters don't change what word it is) and punctuation. Assume that all words in the topic list are already lowercased and do not contain punctuation.
Sure, here's an implementation in Python:
```python
import re
def about(topic):
# compile a regular expression to match any of the topic words
pattern = re.compile("|".join(re.escape(word) for word in topic), re.IGNORECASE)
def contains_topic(paragraph):
# remove all punctuation from the paragraph
paragraph = re.sub(r"\p{P}+", "", paragraph)
# check if the paragraph contains any of the topic words
return bool(pattern.search(paragraph))
return contains_topic
```
Here's an explanation of how it works:
- `re.compile("|".join(re.escape(word) for word in topic), re.IGNORECASE)` creates a regular expression that matches any of the topic words, ignoring case.
- `def contains_topic(paragraph)` defines a function that takes a paragraph and checks if it contains any of the topic words.
- `re.sub(r"\p{P}+", "", paragraph)` removes all punctuation from the paragraph using a regular expression.
- `bool(pattern.search(paragraph))` checks if any of the topic words match the paragraph, returning `True` if there is a match and `False` otherwise.
- `return contains_topic` returns the function that checks for topic words in a paragraph.
To use it, you could create a list of topic words and pass it to `about` to create a function that checks for those words:
```python
topic = ["python", "programming", "code"]
contains_topic = about(topic)
paragraph = "I love programming in Python. Writing code is so much fun!"
if contains_topic(paragraph):
print("This paragraph is about programming!")
else:
print("This paragraph is not about programming.")
```
Output:
```
This paragraph is about programming!
```
Binary search is a common search approach for a list of values. For binary search to work, the elements in the array must already be ordered. Assume that the array is in ascending order. The binary search first compares the key with the element in the middle of the array. Consider the following three cases: ■ If the key is less than the middle element, you need to continue to search for the key only in the first half of the array. ■ If the key is equal to the middle element, the search ends with a match. ■ If the key is greater than the middle element, you need to continue to search for the key only in the second half of the array. Implement the binary search using Language C. And write a program to test whether it can work or not.
Here is a sample implementation of binary search in C:
```
#include <stdio.h>
int binarySearch(int arr[], int left, int right, int key) {
while (left <= right) {
int mid = (left + right) / 2;
if (arr[mid] == key) {
return mid;
}
else if (arr[mid] < key) {
left = mid + 1;
}
else {
right = mid - 1;
}
}
return -1;
}
int main() {
int arr[] = { 2, 4, 6, 8, 10, 12, 14, 16, 18, 20 };
int n = sizeof(arr) / sizeof(arr[0]);
int key = 12;
int index = binarySearch(arr, 0, n - 1, key);
if (index == -1) {
printf("Element not found\n");
}
else {
printf("Element found at index %d\n", index);
}
return 0;
}
```
In this implementation, `binarySearch()` takes four arguments: the array to search `arr`, the left and right indices of the subarray to search `left` and `right`, and the key to search for `key`. It returns the index of the key in the array if it is found, or `-1` if it is not found.
The function works by repeatedly dividing the subarray in half using the middle index `mid`, comparing the key to the element at `arr[mid]`, and adjusting the left and right indices accordingly until the key is found or the subarray is exhausted.
In the `main()` function, we test the `binarySearch()` function by searching for the key `12` in the array `arr`. If the key is found, we print its index; otherwise, we print a message indicating that the element was not found.
阅读全文
相关推荐
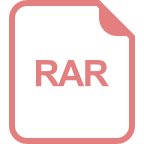
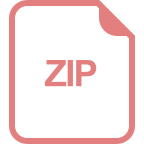
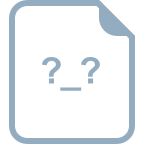












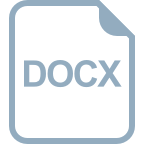