MFC C++ 代码实现异步RS422 串口 发送和接收数据
时间: 2024-10-08 14:05:20 浏览: 10
MFC (Microsoft Foundation Classes) 是 Microsoft Visual Studio 中用于创建 Windows 应用程序的一种框架,它结合了 C++ 和 COM 技术。在 C++ 中通过 MFC 实现异步 RS-422 串口通信,通常会用到 `CSerialPort` 类(如果你使用的是 MFC 的 Serial Port 功能),以及 Win32 API 中的 `CreateThread` 函数来处理串口操作的独立线程。
以下是一个简单的示例,展示如何在 MFC 中发送和接收异步 RS-422 数据:
```cpp
#include "stdafx.h"
#include "resource.h"
#include <afxwin.h>
#include <atlaseserial.h>
// 假设有一个名为 CMyApp 的应用程序
class CMyApp : public CWinApp {
public:
// 初始化串口
void InitializeSerialPort() {
CDC* pDC = new CDC();
CSleepSerial port(pDC);
if (!port.Open(_T("COM1"), CBR_9600, PARITY_NONE, 8, STOPBITS_ONE)) {
AfxMessageBox("Failed to open serial port!");
delete pDC;
return;
}
// 设置为异步模式
port.SetMode(SerialPort::MODE_ASYNC);
// 开始监听接收线程
pDC->StartReceiveThread();
}
// 异步发送数据函数
void SendData(const char* data, int length) {
port.Write(data, length);
}
// 收取接收到的数据(这里只是一个基本示例,实际应用需要处理缓冲区)
void ReceiveData() {
static char buffer[1024];
int received = port.Read(buffer, sizeof(buffer));
if (received > 0) {
AfxMessageBox(std::string(buffer, received).c_str());
} else {
AfxMessageBox("No data received.");
}
}
private:
// 省略其他代码...
};
int WINAPI _tWinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPTSTR lpCmdLine, int nCmdShow) {
CMyApp theApp;
theApp.InitializeSerialPort(); // 初始化串口并启动接收线程
// 然后你可以异步发送数据
theApp.SendData("Hello, RS-422!", strlen("Hello, RS-422!"));
// 在需要的时候接收数据
theApp.ReceiveData();
return theApp.Run();
}
```
相关推荐
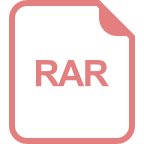
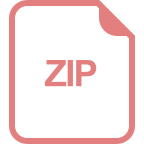
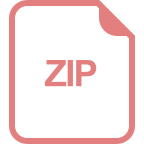














