stm32 按键控制灯光亮度代码
时间: 2023-09-05 19:08:30 浏览: 160
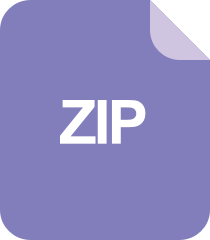
STM32 通过按键控制LED灯亮度

以下是一个简单的示例代码,实现了通过按键控制LED灯的亮度:
```
#include "stm32f10x.h"
#define LED_PIN GPIO_Pin_13
#define LED_GPIO_PORT GPIOC
#define LED_GPIO_CLK RCC_APB2Periph_GPIOC
#define KEY_PIN GPIO_Pin_0
#define KEY_GPIO_PORT GPIOA
#define KEY_GPIO_CLK RCC_APB2Periph_GPIOA
uint16_t led_brightness = 0; // 初始亮度为0
void LED_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(LED_GPIO_CLK, ENABLE);
GPIO_InitStructure.GPIO_Pin = LED_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_GPIO_PORT, &GPIO_InitStructure);
}
void KEY_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(KEY_GPIO_CLK, ENABLE);
GPIO_InitStructure.GPIO_Pin = KEY_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(KEY_GPIO_PORT, &GPIO_InitStructure);
}
int main(void)
{
LED_Init();
KEY_Init();
while (1)
{
if (GPIO_ReadInputDataBit(KEY_GPIO_PORT, KEY_PIN) == 0) // 如果按键被按下
{
__delay_ms(10); // 延时10ms,避免按键抖动
if (GPIO_ReadInputDataBit(KEY_GPIO_PORT, KEY_PIN) == 0) // 如果按键仍然被按下
{
led_brightness += 10; // 增加亮度
if (led_brightness > 100)
led_brightness = 0; // 最大亮度为100,超过则变为最暗
}
}
if (led_brightness == 0)
{
GPIO_ResetBits(LED_GPIO_PORT, LED_PIN); // 关灯
}
else
{
uint16_t delay_time = 1000 / led_brightness; // 计算闪烁延时
GPIO_SetBits(LED_GPIO_PORT, LED_PIN); // 开灯
__delay_ms(delay_time);
GPIO_ResetBits(LED_GPIO_PORT, LED_PIN); // 关灯
__delay_ms(delay_time);
}
}
}
```
代码中使用了两个GPIO端口,一个用于控制LED的亮度,一个用于读取按键的状态。按键被按下时,代码会将LED的亮度增加10,最大亮度为100,超过则变为最暗。在主循环中,根据当前的亮度值来闪烁LED灯。
阅读全文
相关推荐
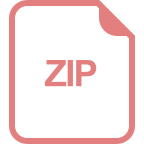
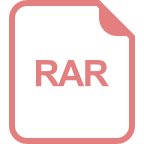
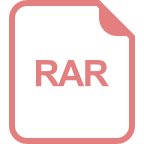





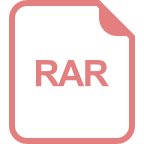
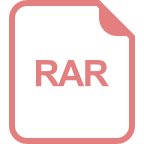
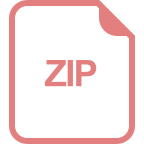
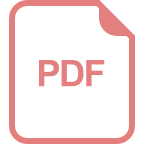
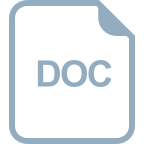
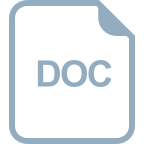
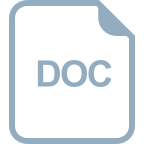

