怎样在@numba.njit修饰的函数中使用functools.partial与numpy.frompyfunc这两个函数
时间: 2024-06-06 11:09:25 浏览: 275
在@numba.njit修饰的函数中使用functools.partial与numpy.frompyfunc这两个函数,需要进行以下步骤:
1. 导入functools和numpy模块。
import functools
import numpy as np
2. 使用functools.partial创建一个新的函数。
partial_func = functools.partial(np.frompyfunc, lambda x: x * 2, 1)
3. 在@numba.njit修饰的函数中使用partial_func。
@numba.njit
def my_func(n):
arr = np.arange(n)
new_arr = partial_func(arr)
return new_arr
这样就可以在@numba.njit修饰的函数中使用functools.partial与numpy.frompyfunc这两个函数了。
相关问题
怎样在numba.jit中使用functools.partial?
在 numba.jit 中使用 functools.partial 非常简单,只需要在使用 functools.partial 生成函数之后将其传递给 numba.jit 即可。例如:
```
from functools import partial
import numba
@numba.jit
def my_func(func, x, y):
return func(x, y)
my_partial_func = partial(my_func, lambda x, y: x + y)
result = my_partial_func(1, 2) # 3
```
在这个例子中,使用 functools.partial 生成了一个新的函数 my_partial_func,它使用 lambda 函数对两个参数进行相加。然后将 my_partial_func 传递给 numba.jit 进行加速,最终通过调用 my_partial_func(1, 2) 得到了结果 3。
def point_cloud_template_matching(template, scene, threshold): """ 点云模板匹配算法 :param template: 模板点云,Nx3的numpy数组 :param scene: 场景点云,Mx3的numpy数组 :param threshold: 匹配阈值 :return: 匹配结果,Nx3的numpy数组,每行表示模板中的一个点在场景中的匹配点 """ # 构建KD树 tree = KDTree(scene) # 查询每个模板点的最近邻 dist, idx = tree.query(template) # 根据阈值筛选匹配点 matches = template[dist < threshold] return matches @numba.njit() def for_prodata(distances, num_samples, farthest_pts, points): for i in range(1, num_samples): # 选择距离已选点集合最远的点 farthest_pts[i] = points[np.argmax(distances)] # 更新距离 distances = np.minimum(distances, np.sqrt(((points - farthest_pts[i]) ** 2).sum(axis=1))) return farthest_pts解释这两段代码
这两段代码是点云模板匹配算法的实现,其中第一段代码实现了匹配过程,第二段代码实现了贪心算法中的迭代过程。
具体解释如下:
第一段代码中,定义了一个名为point_cloud_template_matching的函数,接收三个参数:模板点云template,场景点云scene和匹配阈值threshold。该函数首先使用场景点云构建了一个KD树,然后对于模板点云中的每个点,通过查询KD树找到场景点云中与其距离最近的点,并计算其距离。最后,根据设定的匹配阈值筛选出与模板点云中每个点匹配的场景点云中的点,并将这些点作为匹配结果返回。
第二段代码中,定义了一个名为for_prodata的函数,使用了@numba.njit()装饰器,表示使用Numba进行即时编译,优化代码的运行速度。该函数接收四个参数:距离distances、采样点数目num_samples、已选最远点集合farthest_pts和点云点集points。该函数的作用是在贪心算法中的每一轮迭代中,选择距离已选点集合最远的点,并更新距离。具体实现过程是,首先将第一个采样点作为已选点集合中的第一个点,然后对于每个后续采样点,选择距离已选点集合中的点最远的点作为新的采样点,并更新距离。最终,返回采样点集合farthest_pts。
阅读全文
相关推荐
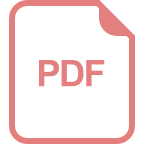
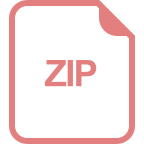
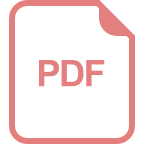












