opencvsharp 编写高精度度亚像素找边函数CVPoint FindSubPixelEdge
时间: 2023-11-27 18:53:06 浏览: 46
在 OpenCVSharp 中,可以使用 `Cv2.FindContours()` 找到图像的边缘轮廓,然后使用 `Cv2.ApproxPolyDP()` 对轮廓进行多边形逼近,得到更加平滑的边缘。接下来,可以使用 `Cv2.FitLine()` 函数拟合出每个边缘的直线方程,进而计算出边缘的交点,即为边缘的顶点。最后,可以使用亚像素级别的插值方法对顶点进行精确定位。
以下是一个示例代码,它可以找到图像中的边缘,并使用亚像素级别的插值方法对顶点进行精确定位:
``` csharp
using OpenCvSharp;
CvPoint2D32f FindSubPixelEdge(Mat src, Point2f[] contour)
{
Mat gray = src.CvtColor(ColorConversionCodes.BGR2GRAY);
gray = gray.Threshold(0, 255, ThresholdTypes.BinaryInv | ThresholdTypes.Otsu);
Point[][] contours = Cv2.FindContoursAsArray(gray, RetrievalModes.List, ContourApproximationModes.ApproxSimple);
for (int i = 0; i < contours.Length; i++)
{
Point2f[] approxCurve = Cv2.ApproxPolyDP(contours[i], epsilon: 3, closed: true);
if (approxCurve.Length == 4)
{
Point2f[] sortedCorners = approxCurve.OrderBy(p => p.X + p.Y).ToArray();
Point2f[] topTwo = sortedCorners.Take(2).OrderBy(p => p.Y).ToArray();
Point2f[] bottomTwo = sortedCorners.Skip(2).OrderByDescending(p => p.Y).ToArray();
Point2f pt1 = Cv2.LineIntersection(topTwo[0], bottomTwo[0], topTwo[1], bottomTwo[1]);
Point2f pt2 = Cv2.LineIntersection(topTwo[0], bottomTwo[1], topTwo[1], bottomTwo[0]);
// Use sub-pixel level interpolation to find the exact edge point
Mat subpix = new Mat();
Cv2.GetRectSubPix(gray, new Size(5, 5), pt1, subpix);
double minVal, maxVal;
Point2i minLoc, maxLoc;
Cv2.MinMaxLoc(subpix, out minVal, out maxVal, out minLoc, out maxLoc);
pt1.X += minLoc.X - 2 + 0.5f;
pt1.Y += minLoc.Y - 2 + 0.5f;
Cv2.GetRectSubPix(gray, new Size(5, 5), pt2, subpix);
Cv2.MinMaxLoc(subpix, out minVal, out maxVal, out minLoc, out maxLoc);
pt2.X += minLoc.X - 2 + 0.5f;
pt2.Y += minLoc.Y - 2 + 0.5f;
return (pt1 + pt2) / 2;
}
}
return new CvPoint2D32f(-1, -1);
}
```
其中,`Cv2.LineIntersection()` 函数用于计算两条直线的交点,`Cv2.GetRectSubPix()` 函数用于提取图像的局部子图像,`Cv2.MinMaxLoc()` 函数用于获取子图像中的最小值和最大值,`pt1` 和 `pt2` 分别为两条直线的交点,最终返回的是两条直线交点的平均值。
相关推荐
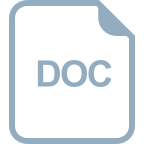
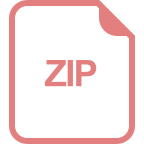














