某班需要班长主持班会,应用单例模式(分别用懒汉式单例和饿汉式单例两种实现形式)产生班长,模拟班会举行
时间: 2023-05-31 10:02:04 浏览: 68
懒汉式单例实现:
```python
class ClassPresident:
__instance = None
@staticmethod
def get_instance():
if ClassPresident.__instance is None:
ClassPresident()
return ClassPresident.__instance
def __init__(self):
if ClassPresident.__instance is not None:
raise Exception("ClassPresident class is a singleton!")
else:
ClassPresident.__instance = self
def lead_class_meeting(self):
print("Welcome to the class meeting. Let's begin!")
# 测试代码
president1 = ClassPresident.get_instance()
president1.lead_class_meeting() # Welcome to the class meeting. Let's begin!
president2 = ClassPresident.get_instance()
print(president1 == president2) # True
president3 = ClassPresident() # 会报错,因为 ClassPresident 类是单例模式,不能直接实例化
```
饿汉式单例实现:
```python
class ClassPresident:
__instance = ClassPresident()
@staticmethod
def get_instance():
return ClassPresident.__instance
def __init__(self):
if ClassPresident.__instance is not None:
raise Exception("ClassPresident class is a singleton!")
def lead_class_meeting(self):
print("Welcome to the class meeting. Let's begin!")
# 测试代码
president1 = ClassPresident.get_instance()
president1.lead_class_meeting() # Welcome to the class meeting. Let's begin!
president2 = ClassPresident.get_instance()
print(president1 == president2) # True
president3 = ClassPresident() # 会报错,因为 ClassPresident 类是单例模式,不能直接实例化
```
模拟班会举行:
```python
president = ClassPresident.get_instance()
president.lead_class_meeting()
# 输出:
# Welcome to the class meeting. Let's begin!
```
相关推荐
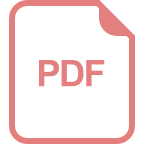














