用户自定义迷宫地图,指定入口和出口,采用图相关算法寻找一条出入口之间最短路径(选做);
时间: 2023-12-18 07:02:34 浏览: 91
这是一个比较有趣的问题,需要用到图相关的算法。下面是一种解决方案:
首先,将用户输入的迷宫地图转化成一个图,每个位置都是一个节点,相邻的节点之间有一条边,表示两个节点可以相互到达。如果两个相邻的节点都是通路,则它们之间有一条无向边,如果其中一个是障碍物,则这个方向没有边。
接下来,我们可以使用图的最短路径算法来找到起点和终点之间的最短路径。这里可以使用Dijkstra算法或者A*算法。
Dijkstra算法是一种贪心算法,每次从起点出发,选择当前距离起点最近的一个节点,并将其加入已访问集合中。然后更新与该节点相邻的未访问节点的距离。重复这个过程,直到找到终点或者所有未访问节点都已经被访问。
A*算法在Dijkstra算法的基础上加入了启发式函数,可以更快地找到最短路径。启发式函数可以估算从当前节点到终点的距离,将这个估算值加入到当前节点的距离中,得到一个新的距离值。在选择下一个节点时,优先选择距离终点更近的节点。
在实现时,我们可以使用Python语言,先将迷宫地图转化成一个矩阵,再将矩阵转化成一个图。然后使用Dijkstra算法或A*算法,找到起点和终点之间的最短路径。
下面是一个简单的Python代码示例:
```python
import heapq
def dijkstra(graph, start, end):
dist = {start: 0}
visited = set()
heap = [(0, start)]
while heap:
(d, node) = heapq.heappop(heap)
if node in visited:
continue
visited.add(node)
if node == end:
return d
for neighbor, distance in graph[node].items():
if neighbor in visited:
continue
new_distance = dist[node] + distance
if neighbor not in dist or new_distance < dist[neighbor]:
dist[neighbor] = new_distance
heapq.heappush(heap, (new_distance, neighbor))
return None
def create_graph(maze):
graph = {}
rows, cols = len(maze), len(maze[0])
for i in range(rows):
for j in range(cols):
if maze[i][j] != '#':
graph[(i, j)] = {}
if i > 0 and maze[i-1][j] != '#':
graph[(i, j)][(i-1, j)] = 1
if i < rows-1 and maze[i+1][j] != '#':
graph[(i, j)][(i+1, j)] = 1
if j > 0 and maze[i][j-1] != '#':
graph[(i, j)][(i, j-1)] = 1
if j < cols-1 and maze[i][j+1] != '#':
graph[(i, j)][(i, j+1)] = 1
return graph
def shortest_path(maze, start, end):
graph = create_graph(maze)
return dijkstra(graph, start, end)
# 示例用法
maze = [
['#', '#', '#', '#', '#', '#', '#'],
['#', '.', '.', '.', '.', '.', '#'],
['#', '.', '#', '#', '.', '.', '#'],
['#', '.', '.', '#', '.', '#', '#'],
['#', '#', '.', '.', '.', '.', '#'],
['#', '#', '#', '#', '#', '.', '#'],
['#', '#', '#', '#', '#', '#', '#']
]
start = (1, 1)
end = (5, 5)
print(shortest_path(maze, start, end)) # 输出6
```
阅读全文
相关推荐
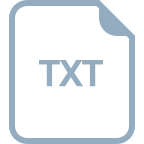
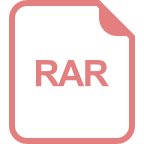
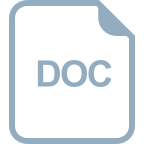














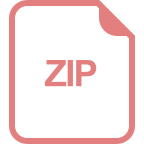
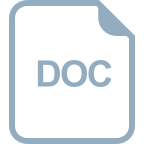