首页我的代码是: import numpy as np from sklearn.preprocessing import PolynomialFeatures # 创建一个随机的输入数据,形状为(10, 3) X = np.random.randn(10, 3) # 创建一个多项式特征转换器,指定最大度数为8,不包含偏置项 poly = PolynomialFeatures(degree=8, include_bias=False) # 对输入数据进行多项式特征转换,得到一个形状为(10, 512)的输出数据 X_poly = poly.fit_transform(X) # 打印输出数据的形状 print(X_poly.shape) 我的输出是: (10, 164)
我的代码是: import numpy as np from sklearn.preprocessing import PolynomialFeatures # 创建一个随机的输入数据,形状为(10, 3) X = np.random.randn(10, 3) # 创建一个多项式特征转换器,指定最大度数为8,不包含偏置项 poly = PolynomialFeatures(degree=8, include_bias=False) # 对输入数据进行多项式特征转换,得到一个形状为(10, 512)的输出数据 X_poly = poly.fit_transform(X) # 打印输出数据的形状 print(X_poly.shape) 我的输出是: (10, 164)
时间: 2024-02-12 15:07:09 浏览: 231
你的代码中指定的最大度数为8,但是输出的数据的第二个维度只有164,这个不够容纳最大度数为8的所有组合特征。你可以检查一下,是否在创建多项式特征转换器时,传入的参数degree不正确。另外,你可以使用下面的代码来检查每个特征的系数,看看是否有问题:
```python
coefs = poly.get_feature_names()
print(coefs)
```
如果输出的coefs中有缺失的特征项,那么说明在特征转换过程中可能出现了问题。
阅读全文
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
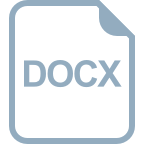
基于java的论坛系统的开题报告.docx
基于java的论坛系统的开题报告
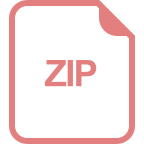
Python中快速友好的MessagePack序列化库msgspec
资源摘要信息:"msgspec是一个针对Python语言的高效且用户友好的MessagePack序列化库。MessagePack是一种快速的二进制序列化格式,它旨在将结构化数据序列化成二进制格式,这样可以比JSON等文本格式更快且更小。msgspec库充分利用了Python的类型提示(type hints),它支持直接从Python类定义中生成序列化和反序列化的模式。对于开发者来说,这意味着使用msgspec时,可以减少手动编码序列化逻辑的工作量,同时保持代码的清晰和易于维护。
msgspec支持Python 3.8及以上版本,能够处理Python原生类型(如int、float、str和bool)以及更复杂的数据结构,如字典、列表、元组和用户定义的类。它还能处理可选字段和默认值,这在很多场景中都非常有用,尤其是当消息格式可能会随着时间发生变化时。
在msgspec中,开发者可以通过定义类来描述数据结构,并通过类继承自`msgspec.Struct`来实现。这样,类的属性就可以直接映射到消息的字段。在序列化时,对象会被转换为MessagePack格式的字节序列;在反序列化时,字节序列可以被转换回原始对象。除了基本的序列化和反序列化,msgspec还支持运行时消息验证,即可以在反序列化时检查消息是否符合预定义的模式。
msgspec的另一个重要特性是它能够处理空集合。例如,上面的例子中`User`类有一个名为`groups`的属性,它的默认值是一个空列表。这种能力意味着开发者不需要为集合中的每个字段编写额外的逻辑,以处理集合为空的情况。
msgspec的使用非常简单直观。例如,创建一个`User`对象并序列化它的代码片段显示了如何定义一个用户类,实例化该类,并将实例序列化为MessagePack格式。这种简洁性是msgspec库的一个主要优势,它减少了代码的复杂性,同时提供了高性能的序列化能力。
msgspec的设计哲学强调了性能和易用性的平衡。它利用了Python的类型提示来简化模式定义和验证的复杂性,同时提供了优化的内部实现来确保快速的序列化和反序列化过程。这种设计使得msgspec非常适合于那些需要高效、类型安全的消息处理的场景,比如网络通信、数据存储以及服务之间的轻量级消息传递。
总的来说,msgspec为Python开发者提供了一个强大的工具集,用于处理高性能的序列化和反序列化任务,特别是当涉及到复杂的对象和结构时。通过利用类型提示和用户定义的模式,msgspec能够简化代码并提高开发效率,同时通过运行时验证确保了数据的正确性。"

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
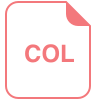
STM32 HAL库函数手册精读:最佳实践与案例分析
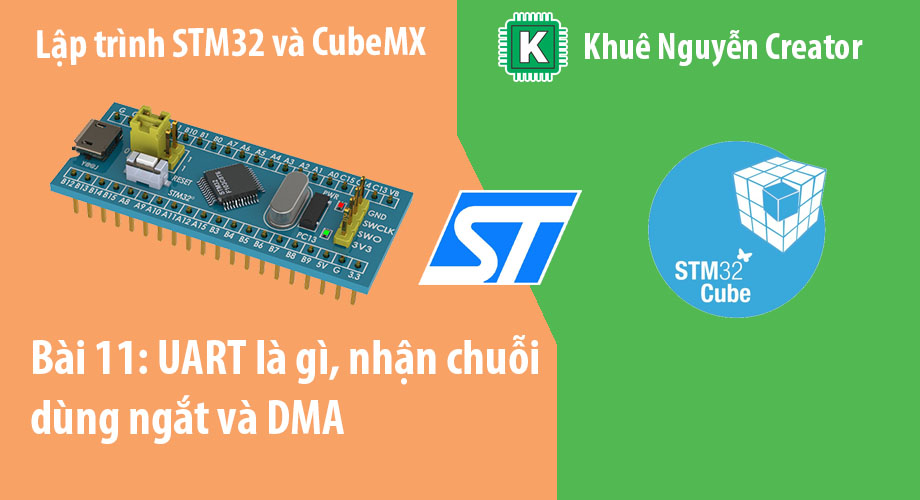
参考资源链接:[STM32CubeMX与STM32HAL库开发者指南](https://wenku.csdn.net/doc/6401ab9dcce7214c316e8df8?spm=1055.2635.3001.10343)
# 1. STM32与HAL库概述
## 1.1 STM32与HAL库的初识
STM32是一系列广泛使用的ARM Cortex-M微控制器,以其高性能、低功耗、丰富的外设接

如何利用FineReport提供的预览模式来优化报表设计,并确保最终用户获得最佳的交互体验?
针对FineReport预览模式的应用,这本《2020 FCRA报表工程师考试题库与答案详解》详细解读了不同预览模式的使用方法和场景,对于优化报表设计尤为关键。首先,设计报表时,建议利用FineReport的分页预览模式来检查报表的布局和排版是否准确,因为分页预览可以模拟报表在打印时的页面效果。其次,通过填报预览模式,可以帮助开发者验证用户交互和数据收集的准确性,这对于填报类型报表尤为重要。数据分析预览模式则适合于数据可视化报表,可以在这个模式下调整数据展示效果和交互设计,确保数据的易读性和分析的准确性。表单预览模式则更多关注于表单的逻辑和用户体验,可以用于检查表单的流程是否合理,以及数据录入
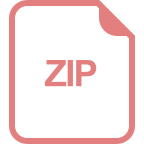
大学生社团管理系统设计与实现
资源摘要信息:"基于ssm+vue的大学生社团管理系统.zip"
该系统是基于Java语言开发的,使用了ssm框架和vue前端框架,主要面向大学生社团进行管理和运营,具备了丰富的功能和良好的用户体验。
首先,ssm框架是Spring、SpringMVC和MyBatis三个框架的整合,其中Spring是一个全面的企业级框架,可以处理企业的业务逻辑,实现对象的依赖注入和事务管理。SpringMVC是基于Servlet API的MVC框架,可以分离视图和模型,简化Web开发。MyBatis是一个支持定制化SQL、存储过程以及高级映射的持久层框架。
SpringBoot是一种全新的构建和部署应用程序的方式,通过使用SpringBoot,可以简化Spring应用的初始搭建以及开发过程。它使用了特定的方式来进行配置,从而使开发人员不再需要定义样板化的配置。
Vue.js是一个用于创建用户界面的渐进式JavaScript框架,它的核心库只关注视图层,易于上手,同时它的生态系统也十分丰富,提供了大量的工具和库。
系统主要功能包括社团信息管理、社团活动管理、社团成员管理、社团财务管理等。社团信息管理可以查看和编辑社团的基本信息,如社团名称、社团简介等;社团活动管理可以查看和编辑社团的活动信息,如活动时间、活动地点等;社团成员管理可以查看和编辑社团成员的信息,如成员姓名、成员角色等;社团财务管理可以查看和编辑社团的财务信息,如收入、支出等。
此外,该系统还可以通过微信小程序进行访问,微信小程序是一种不需要下载安装即可使用的应用,它实现了应用“触手可及”的梦想,用户扫一扫或者搜一下即可打开应用。同时,它也实现了应用“用完即走”的理念,用户不用关心是否安装太多应用的问题。应用将无处不在,随时可用,但又无需安装卸载。
总的来说,基于ssm+vue的大学生社团管理系统是一款功能丰富、操作简便、使用方便的社团管理工具,非常适合大学生社团的日常管理和运营。

"互动学习:行动中的多样性与论文攻读经历"
多样性她- 事实上SCI NCES你的时间表ECOLEDO C Tora SC和NCESPOUR l’Ingén学习互动,互动学习以行动为中心的强化学习学会互动,互动学习,以行动为中心的强化学习计算机科学博士论文于2021年9月28日在Villeneuve d'Asq公开支持马修·瑟林评审团主席法布里斯·勒菲弗尔阿维尼翁大学教授论文指导奥利维尔·皮耶昆谷歌研究教授:智囊团论文联合主任菲利普·普雷教授,大学。里尔/CRISTAL/因里亚报告员奥利维耶·西格德索邦大学报告员卢多维奇·德诺耶教授,Facebook /索邦大学审查员越南圣迈IMT Atlantic高级讲师邀请弗洛里安·斯特鲁布博士,Deepmind对于那些及时看到自己错误的人...3谢谢你首先,我要感谢我的两位博士生导师Olivier和Philippe。奥利维尔,"站在巨人的肩膀上"这句话对你来说完全有意义了。从科学上讲,你知道在这篇论文的(许多)错误中,你是我可以依
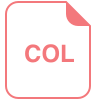
STM32 HAL库深度解析:新手到高手的进阶之路
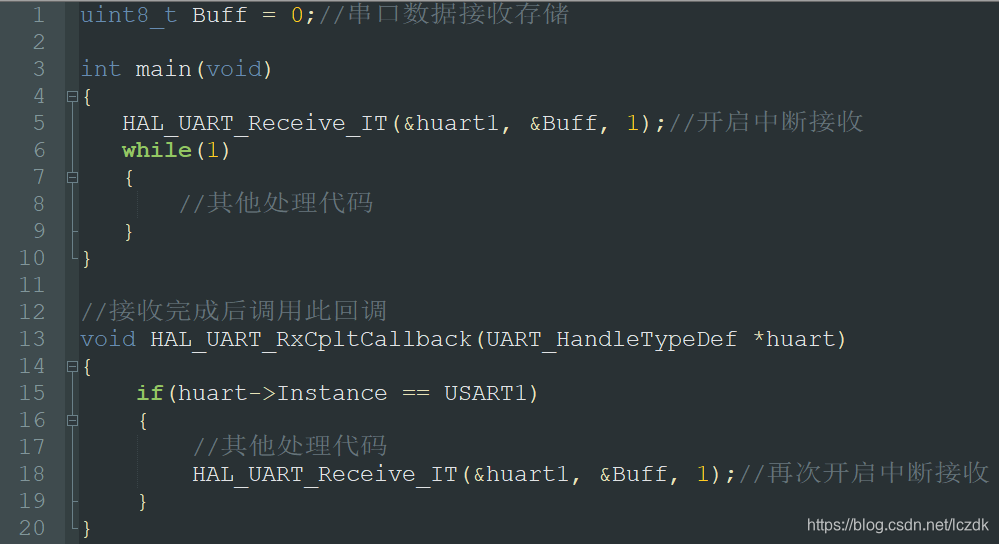
参考资源链接:[STM32CubeMX与STM32HAL库开发者指南](https://wenku.csdn.net/doc/6401ab9dcce7214c316e8df

如何使用pyCUDA库在GPU上进行快速傅里叶变换(FFT)以加速线性代数运算?请提供具体的代码实现。
当你希望利用GPU的并行计算能力来加速线性代数运算,特别是快速傅里叶变换(FFT)时,pyCUDA是一个非常强大的工具。它允许开发者通过Python语言来编写CUDA代码,执行复杂的GPU计算任务。通过学习《Python与pyCUDA:GPU并行计算入门与实战》这一资料,你可以掌握如何使用pyCUDA进行GPU编程和加速计算。
参考资源链接:[Python与pyCUDA:GPU并行计算入门与实战](https://wenku.csdn.net/doc/6401ac00cce7214c316ea46b?spm=1055.2569.3001.10343)
具体到FFT的实现,你需要首先确保已经
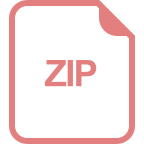
基于Netbeans和JavaFX的宿舍管理系统开发与实践
资源摘要信息:"Hostel-Management-System是一个基于Java技术栈构建的独立应用程序,主要目的是实现一个宿舍管理系统的计算机化。这个系统采用了Netbeans集成开发环境、JavaFX作为前端图形用户界面(GUI)技术、Maven作为项目管理和构建工具、以及MySQL作为后端数据库管理系统。整个系统的设计理念是为大学宿舍提供一个高效、用户友好、跨平台的应用,旨在优化宿舍管理的流程,减少繁琐的文书工作,提高工作效率。
***beans集成开发环境
Netbeans是一个开源的集成开发环境(IDE),它支持多种编程语言,特别是Java。IDE提供了代码编写、调试、项目管理等功能,为开发人员提供了一个全面的开发平台。在这个项目中,Netbeans用于编写Java代码,管理项目结构,以及进行代码的编译、构建和部署。
2. JavaFX技术
JavaFX是Java的官方图形用户界面(GUI)库,用于创建富客户端桌面应用程序。JavaFX提供了一系列的界面控件和强大的图形和媒体支持,使得开发人员可以构建出美观且响应迅速的用户界面。在Hostel-Management-System中,JavaFX负责呈现用户界面,提供交互式的图形界面供学生和员工使用。
3. Maven项目管理工具
Maven是一个项目管理和构建自动化工具,主要用于Java项目。Maven通过一个名为POM(项目对象模型)的文件来管理项目的构建、报告和文档。它支持项目生命周期的管理,提供了一套标准的构建流程,可以处理编译、测试、打包等任务。在本项目中,Maven用于管理项目的依赖关系,自动化构建过程,并确保项目结构的一致性和标准化。
4. MySQL数据库系统
MySQL是一种流行的开源关系型数据库管理系统,它使用结构化查询语言(SQL)进行数据库管理。MySQL支持各种操作系统,并能很好地与Java应用程序集成。在宿舍管理系统中,MySQL负责存储所有学生、员工、房间等信息的数据,确保数据的持久化和可检索性。
5. MVC架构
模型-视图-控制器(MVC)是一种软件设计模式,旨在将应用程序的输入、处理和输出分离成三个互相关联的组件。在Hostel-Management-System中,MVC架构有助于组织代码结构,使得系统的可维护性、可测试性和可扩展性得到增强。模型(Model)负责处理数据和业务逻辑,视图(View)负责展示数据,而控制器(Controller)负责接收用户输入并调用模型和视图组件。
6. 用户友好性和跨平台性
系统的开发理念强调用户友好和跨平台特性。用户友好性意味着系统界面直观易用,操作简单,能够快速响应用户的操作。而跨平台性则是指系统能够在多种操作系统上运行,如Windows、macOS、Linux等,这主要归功于Java的跨平台特性以及JavaFX的支持。
7. 系统模块介绍
该宿舍管理系统主要分为两个用户模块:学生模块和员工模块。学生模块允许学生查看宿舍分配情况、报告问题、支付费用等;员工模块则提供给宿舍管理员和会计人员,用于维护学生和员工的信息、管理房间分配、处理费用等。每个模块都拥有适当的权限和功能,确保了数据的安全性和完整性。
8. 数据库设计
数据库设计是宿舍管理系统中非常关键的一部分。良好的数据库设计可以提高数据处理的效率,保证数据的一致性和完整性。本系统中,数据库需要合理地设计表结构来存储学生、员工、房间和其他相关信息。数据库的设计遵循了关系型数据库的范式,减少了数据冗余,提高了查询效率。
综上所述,Hostel-Management-System是一个结合了现代Java技术栈,特别是Netbeans、JavaFX、Maven和MySQL的宿舍管理软件。它不仅提供了一个高度用户友好的界面,还具备跨平台性和模块化设计,能够有效地帮助大学宿舍管理者处理日常管理任务,提升管理效率和质量。"