用另一种方式表达 public Car(int year, string manufacturer, string model, double price, bool isDrivable = true) { this.year = year; this.manufacturer = manufacturer; this.model = model; this.price = price; this.isDrivable = isDrivable; }
时间: 2024-02-14 07:17:41 浏览: 67
这是一个 C# 中的构造函数,它有五个参数:年份 `year`、制造商 `manufacturer`、型号 `model`、价格 `price` 和可驾驶性 `isDrivable`,其中 `isDrivable` 参数有一个默认值 `true`。构造函数的作用是创建一个名为 `Car` 的对象,并初始化它的属性值。
```
public Car(int year, string manufacturer, string model, double price, bool isDrivable = true)
{
this.year = year;
this.manufacturer = manufacturer;
this.model = model;
this.price = price;
this.isDrivable = isDrivable;
}
```
在这个构造函数中,`this.` 表示当前对象的属性值,通过参数传递的值将被赋值给相应的属性。如果 `isDrivable` 参数没有传值,则默认为 `true`。
相关问题
public Car(int year, string manufacturer, string model, double price, bool isDrivable = true) { this.year = year; this.manufacturer = manufacturer; this.model = model; this.price = price; this.isDrivable = isDrivable; }解释以上代码
以上代码是一个名为"Car"的类中的构造函数。它接受5个参数:year,manufacturer,model,price和isDrivable,并将它们分别赋值给类中对应的属性。
其中,isDrivable是一个可选参数,其默认值为true。这意味着,如果在创建Car对象时没有指定isDrivable的值,则它将默认为true。
该构造函数的作用是在创建Car对象时,将传入的参数值分别赋值给相应的属性。例如,如果我们创建一个名为"myCar"的Car对象,代码可能如下所示:
```csharp
Car myCar = new Car(2021, "Toyota", "Corolla", 25000.0);
```
在上面的代码中,我们没有指定isDrivable的值,因此它将默认为true。这将创建一个名为"myCar"的Car对象,将2021、"Toyota"、"Corolla"和25000.0分别赋值给year、manufacturer、model和price属性,并将isDrivable属性的值设置为true。
总之,以上代码是一个用于创建Car对象的构造函数,它接受5个参数,并将它们分别赋值给类中对应的属性。其中,isDrivable是一个可选参数,如果未指定其值,则默认为true。
Use c # to complete the following code,Creating a Car class A class is a self-contained piece of software that is able to function on it own. We will model a car in software. Car Class Properties Year : int «private set» Manufacturer : string «private set» Model : string «private set» IsDrivable : bool «private set» Price : double «private set» Methods«constructor» Car(year : int, manufacturer : string, model : string, price : double, isDrivable: bool = true) PrintInfo() : void Description of field members: 1. year: this private member represents the year value of this object 2. manufacturer: this private member represents the manufacturer of this car 3. model: this private member represents the model of this car 4. isDrivable: this private member indicates if this car be driven 5. price: this private member represents the selling price of this car Constructor: 1. public Car(int year, string manufacturer, string model, double price, bool isDrivable = true): this is the constructor of this class. It takes five arguments and assigns them to the appropriate fields. You will assume that all the arguments will be sane (i.e. the values will not break your program). Apology: UML and some newer languages specify the type after the identifier. C# on the other hand requires that you specify the type and then the identifier
Sure, here is the completed code:
```
public class Car
{
public int Year { get; private set; }
public string Manufacturer { get; private set; }
public string Model { get; private set; }
public bool IsDrivable { get; private set; }
public double Price { get; private set; }
public Car(int year, string manufacturer, string model, double price, bool isDrivable = true)
{
Year = year;
Manufacturer = manufacturer;
Model = model;
Price = price;
IsDrivable = isDrivable;
}
public void PrintInfo()
{
Console.WriteLine($"Year: {Year}, Manufacturer: {Manufacturer}, Model: {Model}, IsDrivable: {IsDrivable}, Price: {Price}");
}
}
```
This code defines a Car class with the specified properties and methods. The constructor takes in the necessary arguments to initialize the object, and the PrintInfo method prints out the information about the car object.
阅读全文
相关推荐
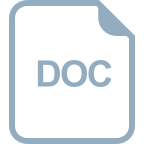
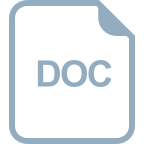
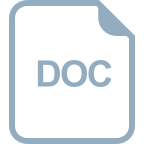



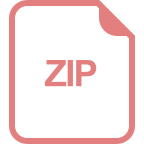
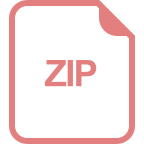
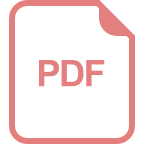
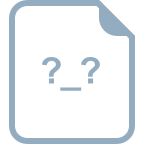
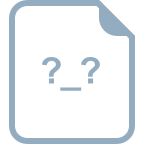
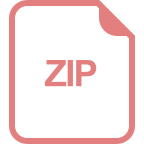
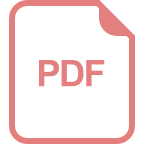
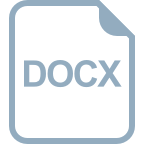
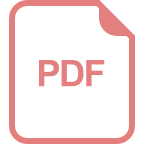
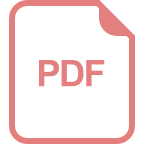
