帮我用c++写一个马踏棋盘代码
时间: 2023-08-28 11:11:04 浏览: 147
### 回答1:
好的,以下是用C语言编写的马踏棋盘代码:
```
#include <stdio.h>
#define N 8
int chessboard[N][N] = {0};
int dx[8] = {-1, -2, -2, -1, 1, 2, 2, 1};
int dy[8] = {-2, -1, 1, 2, 2, 1, -1, -2};
void print_chessboard() {
for(int i = 0; i < N; i++) {
for(int j = 0; j < N; j++) {
printf("%2d ", chessboard[i][j]);
}
printf("\n");
}
}
int can_move(int x, int y) {
if(x < 0 || x >= N || y < 0 || y >= N || chessboard[x][y] != 0) {
return 0;
}
return 1;
}
int solve(int x, int y, int count) {
chessboard[x][y] = count;
if(count == N*N) {
return 1;
}
for(int i = 0; i < 8; i++) {
int new_x = x + dx[i];
int new_y = y + dy[i];
if(can_move(new_x, new_y)) {
if(solve(new_x, new_y, count+1)) {
return 1;
}
}
}
chessboard[x][y] = 0;
return 0;
}
int main() {
int start_x, start_y;
printf("请输入马的起始位置(行 列, 范围为0到7): ");
scanf("%d %d", &start_x, &start_y);
if(can_move(start_x, start_y)) {
solve(start_x, start_y, 1);
print_chessboard();
}
else {
printf("输入的位置不合法!\n");
}
return 0;
}
```
该代码实现了马踏棋盘问题的求解,可以根据用户输入的马的起始位置,在棋盘上输出马的行走路径,其中数字表示马在该位置的步数。
### 回答2:
下面是一段使用C语言编写的马踏棋盘代码:
```c
#include<stdio.h>
#define N 8
int solveKT(int x, int y, int movei, int sol[N][N], int xMove[N], int yMove[N]);
// 检查当前位置是否是合法的步数
int isSafe(int x, int y, int sol[N][N])
{
return (x >=0 && x < N && y >= 0 && y < N && sol[x][y] == -1);
}
// 打印最终的解决方案
void printSolution(int sol[N][N])
{
for (int x = 0; x < N; x++)
{
for(int y = 0; y < N; y++)
{
printf(" %2d ", sol[x][y]);
}
printf("\n");
}
}
// 包含所有可能的骑士移动
int solveKT()
{
int sol[N][N];
// 初始化棋盘
for(int x = 0; x < N; x++)
{
for(int y = 0; y < N; y++)
{
sol[x][y] = -1;
}
}
// 定义骑士的八个可能移动方向
int xMove[8] = {2, 1, -1, -2, -2, -1, 1, 2};
int yMove[8] = {1, 2, 2, 1, -1, -2, -2, -1};
// 初始位置
sol[0][0] = 0;
// 启动递归函数
if(solveKT(0, 0, 1, sol, xMove, yMove) == 0)
{
printf("没有找到路径\n");
return 0;
}
else
{
printf("找到路径:\n");
printSolution(sol);
}
return 1;
}
// 递归函数,使用“回溯”方法找到路径
int solveKT(int x, int y, int movei, int sol[N][N], int xMove[N], int yMove[N])
{
int k, next_x, next_y;
if (movei == N*N)
{
return 1;
}
// 尝试骑士的下一个移动
for (k = 0; k < 8; k++)
{
next_x = x + xMove[k];
next_y = y + yMove[k];
if (isSafe(next_x, next_y, sol))
{
sol[next_x][next_y] = movei;
if (solveKT(next_x, next_y, movei+1, sol, xMove, yMove) == 1)
{
return 1;
}
else
{
sol[next_x][next_y] = -1;
}
}
}
return 0;
}
int main()
{
solveKT();
return 0;
}
```
这段代码使用回溯法来解决“马踏棋盘”问题。它会找出一个骑士可以按照规则依次访问棋盘上所有格子的路径,并在输出中打印出最后的解决方案。
### 回答3:
马踏棋盘是一种经典的数学问题,可以使用回溯算法来解决。以下是一个简单的用C语言编写的马踏棋盘代码:
```c
#include<stdio.h>
#include<stdlib.h>
#define SIZE 5 // 棋盘大小
#define START_ROW 0 // 马的初始位置
#define START_COL 0
int chessboard[SIZE][SIZE]; // 棋盘
int moves[SIZE][SIZE]; // 马的移动路径
int dx[] = {2, 1, -1, -2, -2, -1, 1, 2}; // 马的横向移动方式
int dy[] = {1, 2, 2, 1, -1, -2, -2, -1}; // 马的纵向移动方式
// 判断当前位置是否在棋盘内
int isValidMove(int x, int y) {
if (x >= 0 && x < SIZE && y >= 0 && y < SIZE && chessboard[x][y] == -1) {
return 1;
}
return 0;
}
// 按照马在棋盘上的路径进行移动
int solveKnightTour(int x, int y, int moveCount) {
int k, nextX, nextY;
// 棋盘上的所有位置都被访问过
if (moveCount == SIZE * SIZE) {
return 1;
}
for (k = 0; k < 8; k++) {
nextX = x + dx[k];
nextY = y + dy[k];
if (isValidMove(nextX, nextY)) {
chessboard[nextX][nextY] = moveCount;
moves[nextX][nextY] = moveCount;
if (solveKnightTour(nextX, nextY, moveCount + 1)) {
return 1;
}
chessboard[nextX][nextY] = -1; // 回溯
moves[nextX][nextY] = -1;
}
}
return 0;
}
// 打印棋盘上的移动路径
void printChessboard() {
int i, j;
for (i = 0; i < SIZE; i++) {
for (j = 0; j < SIZE; j++) {
printf("%2d ", moves[i][j]);
}
printf("\n");
}
}
int main() {
int i, j;
// 初始化棋盘和移动路径
for (i = 0; i < SIZE; i++) {
for (j = 0; j < SIZE; j++) {
chessboard[i][j] = -1;
moves[i][j] = -1;
}
}
chessboard[START_ROW][START_COL] = 0;
moves[START_ROW][START_COL] = 0;
// 开始解决马踏棋盘问题
if (solveKnightTour(START_ROW, START_COL, 1)) {
printf("找到一种解决方案:\n");
printChessboard();
}
else {
printf("没有找到解决方案。\n");
}
return 0;
}
```
运行该代码,即可得到马在棋盘上的一种移动路径。代码中使用了回溯算法,通过尝试每一种可能的移动,来找到解决方案。
阅读全文
相关推荐
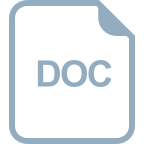
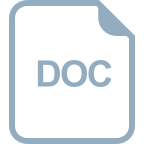
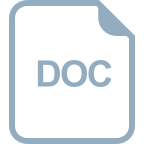

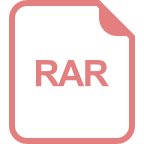
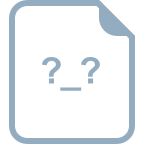
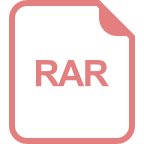
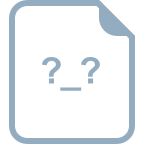
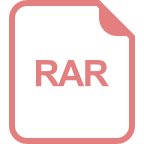
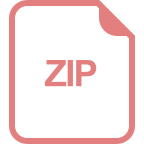
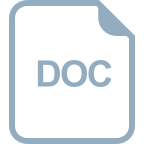
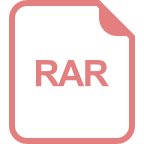
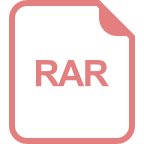
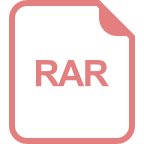
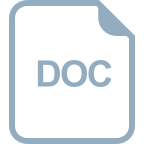
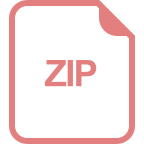
