蛮力法马踏棋盘c++代码
时间: 2024-06-22 20:02:36 浏览: 7
蛮力法,也称为暴力搜索或穷举法,是一种在搜索问题中尝试所有可能解的方法,通常用于解决一些较小规模的问题,如马踏棋盘问题。在C++中,我们可以编写代码模拟马可罗夫走遍所有可能的步数来寻找最短路径。下面是一个简单的马踏棋盘(骑士巡游)的C++代码示例:
```cpp
#include <iostream>
#include <vector>
const int N = 8; // 假设棋盘为8x8
int chessboard[N][N];
// 检查是否合法移动
bool isValid(int x, int y) {
return (x >= 0 && x < N && y >= 0 && y < N && (chessboard[x][y] == 0 || chessboard[x + 2][y + 1] == 0 || // 骑士的两种移动方式
chessboard[x + 1][y + 2] == 0 || chessboard[x - 2][y + 1] == 0 ||
chessboard[x - 1][y + 2] == 0 || chessboard[x - 2][y - 1] == 0 ||
chessboard[x - 1][y - 2] == 0 || chessboard[x + 2][y - 1] == 0));
}
// 蛮力法遍历所有可能的步数
void bruteForce(int steps, int start_x, int start_y) {
if (steps >= N * N) { // 如果步数超过棋盘大小,说明不可能完成,返回false
return;
}
chessboard[start_x][start_y] = steps; // 标记当前位置
for (int dx = -2; dx <= 2; dx++) { // 骑士的四个移动方向
for (int dy = -2; dy <= 2; dy++) {
int new_x = start_x + dx;
int new_y = start_y + dy;
if (isValid(new_x, new_y)) {
bruteForce(steps + 1, new_x, new_y);
}
}
}
}
int main() {
// 初始化棋盘
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
chessboard[i][j] = 0;
}
}
// 搜索起始位置
bruteForce(0, 0, 0); // 假设起始位置是左上角
// 打印最短路径
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
std::cout << chessboard[i][j] << " ";
}
std::cout << "\n";
}
return 0;
}
```
相关推荐
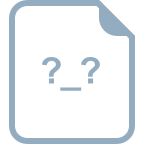
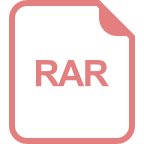














