用c语言写一个完整的马踏棋盘算法
时间: 2023-08-31 21:34:32 浏览: 171
### 回答1:
你好,以下是用 C 语言编写的马踏棋盘算法:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 8
int board[MAX_SIZE][MAX_SIZE];
int move_x[8] = {1, 2, 2, 1, -1, -2, -2, -1};
int move_y[8] = {2, 1, -1, -2, -2, -1, 1, 2};
void print_board() {
for (int i = ; i < MAX_SIZE; i++) {
for (int j = ; j < MAX_SIZE; j++) {
printf("%2d ", board[i][j]);
}
printf("\n");
}
}
int is_valid_move(int x, int y) {
if (x < || x >= MAX_SIZE || y < || y >= MAX_SIZE) {
return ;
}
if (board[x][y] != ) {
return ;
}
return 1;
}
int solve(int x, int y, int step) {
board[x][y] = step;
if (step == MAX_SIZE * MAX_SIZE) {
return 1;
}
for (int i = ; i < 8; i++) {
int next_x = x + move_x[i];
int next_y = y + move_y[i];
if (is_valid_move(next_x, next_y)) {
if (solve(next_x, next_y, step + 1)) {
return 1;
}
}
}
board[x][y] = ;
return ;
}
int main() {
memset(board, , sizeof(board));
if (solve(, , 1)) {
printf("Solution found:\n");
print_board();
} else {
printf("No solution found.\n");
}
return ;
}
希望能对你有所帮助。
### 回答2:
马踏棋盘是一种著名的递归算法,主要用于解决如何使马在棋盘上每个格子只经过一次的问题。下面是使用C语言编写的一个完整的马踏棋盘算法:
```c
#include <stdio.h>
#define SIZE 8 // 棋盘大小
// 马在棋盘上的移动方向和下一个位置的坐标
int nextX[8] = {1, 2, 2, 1, -1, -2, -2, -1};
int nextY[8] = {2, 1, -1, -2, -2, -1, 1, 2};
// 棋盘
int chessboard[SIZE][SIZE];
// 检查下一个位置是否合法
int isLegal(int x, int y) {
if (x >= 0 && x < SIZE && y >= 0 && y < SIZE && chessboard[x][y] == 0) {
return 1;
}
return 0;
}
// 输出棋盘
void printChessboard() {
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
printf("%2d ", chessboard[i][j]);
}
printf("\n");
}
}
// 马踏棋盘算法
int horseStep(int x, int y, int step) {
int nextXPos, nextYPos;
if (step == SIZE * SIZE) { // 遍历完成
printChessboard();
return 1;
}
for (int i = 0; i < 8; i++) { // 尝试八个方向移动
nextXPos = x + nextX[i];
nextYPos = y + nextY[i];
if (isLegal(nextXPos, nextYPos)) {
chessboard[nextXPos][nextYPos] = step;
if (horseStep(nextXPos, nextYPos, step + 1)) { // 递归
return 1;
}
chessboard[nextXPos][nextYPos] = 0; // 回溯
}
}
return 0;
}
int main() {
int startX = 0, startY = 0; // 设置起始位置
chessboard[startX][startY] = 1;
if (!horseStep(startX, startY, 2)) { // 从起始位置开始遍历
printf("无解!\n");
}
return 0;
}
```
以上的代码使用了递归的方式实现了马踏棋盘算法。在检查下一个位置是否合法的时候,判断条件是坐标是否在棋盘内以及该位置是否已经被访问过。在遍历每一个方向的时候,将下一个位置的步数设置为当前步数加一,并递归地调用马踏棋盘函数。如果得到的结果为1,表示遍历完成,打印棋盘;如果结果为0,表示无解。
### 回答3:
马踏棋盘问题是一个经典的回溯算法问题,可以使用C语言编写一个完整的算法来解决。
首先,我们需要定义棋盘的大小和马的初始位置。假设棋盘为8x8,初始位置为(0,0)。
然后,我们需要定义一个二维数组来表示棋盘,并将其初始化为-1,表示未走过。接下来,我们将起始位置设置为0,并从起始位置开始递归遍历棋盘。
在每个位置上,我们首先判断该位置是否越界或者已经被访问过,如果是,则返回False。否则,将该位置标记为当前步数,并且检查是否已经访问了棋盘上的每个位置(即步数是否等于棋盘大小)。
如果是,则打印出结果矩阵,并返回True。否则,我们需要按照马的移动规则进行遍历。马的移动规则是根据马字型的移动方式:横向或纵向移动2步,然后再垂直或水平移动1步。
我们将递归调用该函数来遍历下一个位置,直到找到解决方案或者遍历所有可能的路径。
最后,我们将解决方案编写成一个函数,调用该函数即可得到解决结果。
以下是一个用C语言写的完整的马踏棋盘算法的示例代码:
```c
#include <stdio.h>
#define SIZE 8
int board[SIZE][SIZE];
int moveX[8] = {1, 1, 2, 2, -1, -1, -2, -2};
int moveY[8] = {2, -2, 1, -1, 2, -2, 1, -1};
int solve(int x, int y, int step) {
if (step == SIZE*SIZE) {
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
printf("%2d ", board[i][j]);
}
printf("\n");
}
return 1;
}
for (int i = 0; i < 8; i++) {
int nextX = x + moveX[i];
int nextY = y + moveY[i];
if (nextX >= 0 && nextX < SIZE && nextY >= 0 && nextY < SIZE && board[nextX][nextY] == -1) {
board[nextX][nextY] = step;
if (solve(nextX, nextY, step+1)) {
return 1;
}
board[nextX][nextY] = -1;
}
}
return 0;
}
int main() {
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
board[i][j] = -1;
}
}
board[0][0] = 0;
solve(0, 0, 1);
return 0;
}
```
以上代码可以在一个8x8的棋盘上找到马踏棋盘的一条路径,并将结果输出到终端上。请注意,这个代码只会找到一条路径,可能不是唯一的解决方案。要找到所有解决方案,您可以进行一些修改来支持多个解决方案的记录和输出。
阅读全文
相关推荐
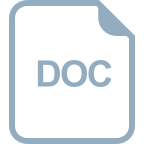
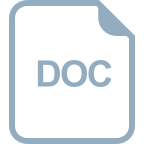
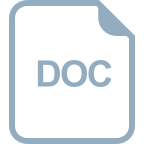
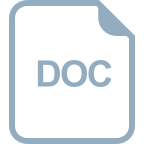
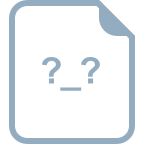
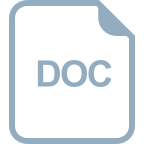
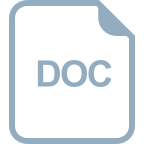
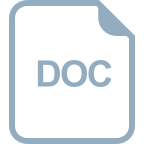
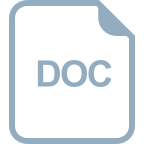
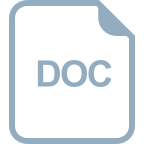
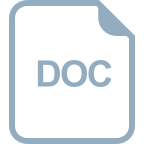

