棋盘覆盖c++全部代码
时间: 2024-04-07 20:25:42 浏览: 112
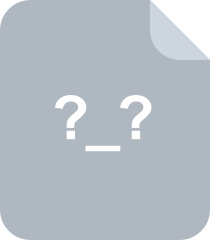
C++棋盘覆盖

棋盘覆盖问题是一个经典的分治算法问题,其主要思想是将一个棋盘划分为若干个子棋盘,然后递归地对每个子棋盘进行覆盖。以下是一个C++的棋盘覆盖问题的完整代码示例[^1][^2]:
```cpp
#include <iostream>
#include <vector>
using namespace std;
// 定义棋盘大小
const int BOARD_SIZE = 8;
// 定义特殊方格的坐标
int specialRow, specialCol;
// 定义骨牌编号
int tile = 1;
// 棋盘覆盖函数
void chessboard(vector<vector<int>>& board, int tr, int tc, int dr, int dc, int size) {
if (size == 1) {
return;
}
int t = tile++;
int s = size / 2;
// 特殊方格在左上棋盘
if (specialRow < tr + s && specialCol < tc + s) {
chessboard(board, tr, tc, dr, dc, s);
} else {
// 骨牌覆盖右下方格
board[tr + s - 1][tc + s - 1] = t;
chessboard(board, tr, tc, tr + s - 1, tc + s - 1, s);
}
// 特殊方格在右上棋盘
if (specialRow < tr + s && specialCol >= tc + s) {
chessboard(board, tr, tc + s, dr, dc, s);
} else {
// 骨牌覆盖左下方格
board[tr + s - 1][tc + s] = t;
chessboard(board, tr, tc + s, tr + s - 1, tc + s, s);
}
// 特殊方格在左下棋盘
if (specialRow >= tr + s && specialCol < tc + s) {
chessboard(board, tr + s, tc, dr, dc, s);
} else {
// 骨牌覆盖右上方格
board[tr + s][tc + s - 1] = t;
chessboard(board, tr + s, tc, tr + s, tc + s - 1, s);
}
// 特殊方格在右下棋盘
if (specialRow >= tr + s && specialCol >= tc + s) {
chessboard(board, tr + s, tc + s, dr, dc, s);
} else {
// 骨牌覆盖左上方格
board[tr + s][tc + s] = t;
chessboard(board, tr + s, tc + s, tr + s, tc + s, s);
}
}
int main() {
// 初始化棋盘
vector<vector<int>> board(BOARD_SIZE, vector<int>(BOARD_SIZE, 0));
// 输入特殊方格的坐标
cout << "Enter the row and column of the special square: ";
cin >> specialRow >> specialCol;
// 调用棋盘覆盖函数
chessboard(board, 0, 0, specialRow, specialCol, BOARD_SIZE);
// 输出棋盘
cout << "The chessboard after covering:" << endl;
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
cout << board[i][j] << "\t";
}
cout << endl;
}
return 0;
}
```
这段代码实现了棋盘覆盖问题的解决方案。首先,通过输入特殊方格的坐标,然后调用`chessboard`函数进行棋盘覆盖。最后,输出覆盖后的棋盘。
阅读全文
相关推荐
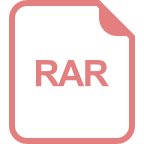
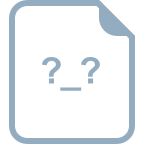
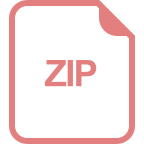
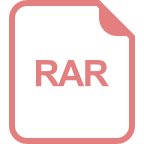
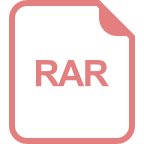
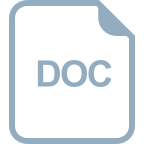









