C++中使用类来实现链表的构造的增删改查
时间: 2024-01-08 16:04:11 浏览: 77
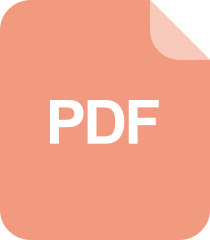
关于双向链表的增删改查和排序的C++实现
下面是用类实现链表的增删改查的示例代码:
```c
#include <stdio.h>
class Node {
public:
int data;
Node* next;
Node(int data) {
this->data = data;
this->next = NULL;
}
};
class LinkedList {
public:
Node* head;
LinkedList() {
this->head = NULL;
}
// 添加节点
void addNode(int data) {
Node* node = new Node(data);
if (this->head == NULL) {
this->head = node;
} else {
Node* current = this->head;
while (current->next != NULL) {
current = current->next;
}
current->next = node;
}
}
// 删除节点
void deleteNode(int data) {
if (this->head == NULL) {
return;
}
if (this->head->data == data) {
Node* temp = this->head;
this->head = this->head->next;
delete temp;
return;
}
Node* current = this->head;
while (current->next != NULL && current->next->data != data) {
current = current->next;
}
if (current->next != NULL) {
Node* temp = current->next;
current->next = current->next->next;
delete temp;
}
}
// 修改节点
void modifyNode(int oldData, int newData) {
Node* current = this->head;
while (current != NULL && current->data != oldData) {
current = current->next;
}
if (current != NULL) {
current->data = newData;
}
}
// 查找节点
Node* searchNode(int data) {
Node* current = this->head;
while (current != NULL && current->data != data) {
current = current->next;
}
return current;
}
// 打印链表
void printList() {
Node* current = this->head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
};
int main() {
LinkedList list;
list.addNode(1);
list.addNode(2);
list.addNode(3);
list.addNode(4);
list.addNode(5);
list.printList();
Node* node = list.searchNode(3);
if (node != NULL) {
printf("Found node with data %d\n", node->data);
} else {
printf("Node not found\n");
}
list.modifyNode(3, 6);
list.printList();
list.deleteNode(4);
list.printList();
return 0;
}
```
输出结果为:
```
1 2 3 4 5
Found node with data 3
1 2 6 4 5
1 2 6 5
```
阅读全文
相关推荐
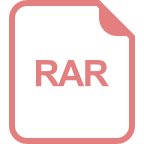
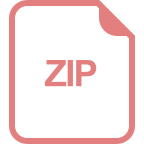












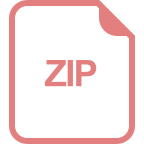
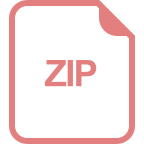
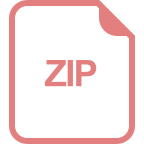
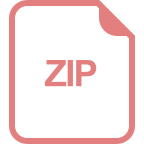