def remove_duplicate_columns(df): df2=df.copy() for i in range(df.shape[1]): for n in range(i+1,df.shape[1]): if df[df.columns[i]].equals([df.columns[n]])==False: continue if df[df.columns[i]].equals([df.columns[n]])==True: del df2[df.columns[i]] return df2 what is wrong with my codes in order to Write a function named remove_duplicate_columns to find the duplicate columns (all values are the same) of a given Pandas DataFrame (all column names are different) and remove the duplicated columns (keep the one that first appears in the DataFrame). The input for the function is a Pandas Dataframe and the output should be a new DataFrame with the duplicate columns removed. For example, if df = pd.DataFrame({'col1': [1, 2, 3], 'col2': [5, 8, 6], 'col3': [7, 9, 8], 'col4': [5, 8, 6]}) then remove_duplicate_columns(df) should return pd.DataFrame({'col1': [1, 2, 3], 'col2': [5, 8, 6], 'col3': [7, 9, 8]), because 'col4' has the same elements as 'col2'. Note that the input should not be modified. (hint: dataframe1.equals(dataframe2) can be used to test whether two dataframes (dataframe1 and dataframe2) contain the same elements).
时间: 2023-11-22 09:57:05 浏览: 156
There are a couple of issues with your code:
1. In the line `if df[df.columns[i]].equals([df.columns[n]])==False:`, you are comparing the values of two columns, but you are wrapping one of them in a list. You should remove the square brackets around `df.columns[n]`.
2. You are deleting columns from `df2` inside the loop, which can cause problems with the indexing. It's better to create a list of columns to delete and then delete them outside the loop.
Here is a corrected version of your code:
```
def remove_duplicate_columns(df):
df2 = df.copy()
columns_to_delete = []
for i in range(df.shape[1]):
for n in range(i+1,df.shape[1]):
if df[df.columns[i]].equals(df[df.columns[n]]) == True:
columns_to_delete.append(df.columns[n])
df2 = df2.drop(columns_to_delete, axis=1)
return df2
```
This function should work correctly for the given problem statement.
相关推荐
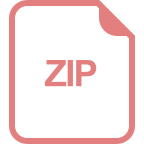
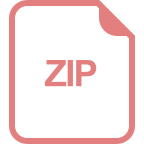
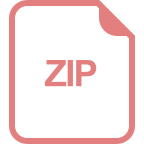
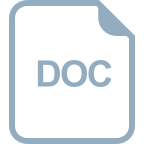
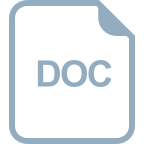
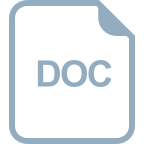
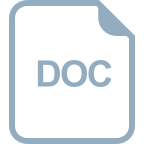
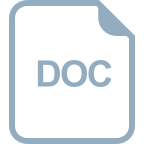
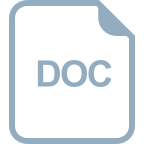
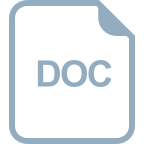
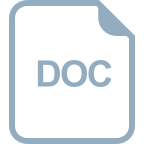
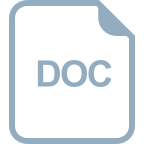
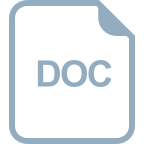
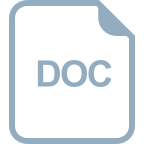
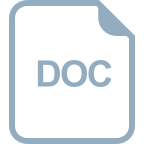
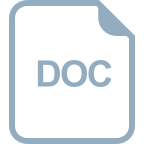
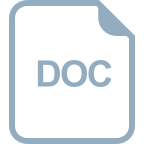
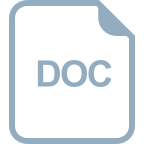
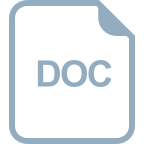