介绍下c++中的ConcurrentHashMap
时间: 2023-10-14 17:26:40 浏览: 60
在C语言中,没有ConcurrentHashMap的内置实现。但是,可以通过使用多线程和锁来实现并发哈希表。下面是一个简单的示例:
```c
#include <pthread.h>
#include <stdbool.h>
#include <stdint.h>
#include <stdlib.h>
#define NUM_BUCKETS 256
typedef struct {
void* key;
void* value;
pthread_mutex_t lock;
} hash_entry_t;
typedef struct {
hash_entry_t* entries;
pthread_mutex_t* locks;
} hash_table_t;
hash_table_t* hash_table_create() {
hash_table_t* table = malloc(sizeof(hash_table_t));
table->entries = calloc(NUM_BUCKETS, sizeof(hash_entry_t));
table->locks = calloc(NUM_BUCKETS, sizeof(pthread_mutex_t));
for (int i = 0; i < NUM_BUCKETS; i++) {
pthread_mutex_init(&table->locks[i], NULL);
}
return table;
}
void hash_table_put(hash_table_t* table, void* key, void* value) {
uint8_t hash = (uint8_t)key % NUM_BUCKETS;
hash_entry_t* entry = &table->entries[hash];
pthread_mutex_t* lock = &table->locks[hash];
pthread_mutex_lock(lock);
if (entry->key == NULL) {
entry->key = key;
entry->value = value;
} else {
while (true) {
if (entry->key == key) {
entry->value = value;
break;
} else if (entry->key == NULL) {
entry->key = key;
entry->value = value;
break;
} else {
hash = (hash + 1) % NUM_BUCKETS;
entry = &table->entries[hash];
lock = &table->locks[hash];
pthread_mutex_lock(lock);
}
}
}
pthread_mutex_unlock(lock);
}
void* hash_table_get(hash_table_t* table, void* key) {
uint8_t hash = (uint8_t)key % NUM_BUCKETS;
hash_entry_t* entry = &table->entries[hash];
pthread_mutex_t* lock = &table->locks[hash];
pthread_mutex_lock(lock);
while (entry->key != NULL && entry->key != key) {
hash = (hash + 1) % NUM_BUCKETS;
entry = &table->entries[hash];
lock = &table->locks[hash];
pthread_mutex_lock(lock);
}
pthread_mutex_unlock(lock);
if (entry->key == NULL) {
return NULL;
} else {
return entry->value;
}
}
void hash_table_destroy(hash_table_t* table) {
for (int i = 0; i < NUM_BUCKETS; i++) {
pthread_mutex_destroy(&table->locks[i]);
}
free(table->entries);
free(table->locks);
free(table);
}
```
在这个实现中,哈希表被分成了多个bucket,每个bucket都有一个锁来确保线程安全。当向哈希表中添加或获取元素时,线程会锁定相应的bucket,以防止其他线程同时访问它。虽然这个实现可能比Java或其他语言中的ConcurrentHashMap更慢,但它仍然是一个可行的并发哈希表实现。
阅读全文
相关推荐



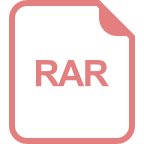




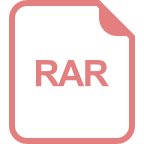









