基于numpy 和 pandas从零开始实现(小批量随机梯度下降法)
时间: 2024-09-12 12:14:23 浏览: 53
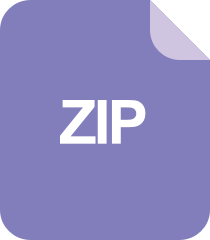
基于梯度下降法的线性回归模型预测房价

基于NumPy和Pandas库,我们可以从零开始实现小批量随机梯度下降法(Stochastic Gradient Descent,SGD),它是一种常用的优化算法,用于训练机器学习模型。这里是一个简化版的示例,我们将创建一个线性回归模型:
```python
import numpy as np
import pandas as pd
# 假设我们有一个简单的数据集
data = {'x': [1, 2, 3, 4, 5], 'y': [2, 4, 6, 8, 10]}
df = pd.DataFrame(data)
# 定义模型参数
weights = np.array([1.0, 1.0]) # 权重向量
learning_rate = 0.01 # 学习率
epochs = 1000 # 迭代次数
def linear_regression(x, y):
return np.dot(x, weights)
def compute_gradient(x, y, prediction):
return - (1 / len(x)) * (x.T @ (prediction - y))
def sgd_step(x, y, weights, learning_rate):
gradient = compute_gradient(x, y, linear_regression(x, y))
weights -= learning_rate * gradient
return weights
for _ in range(epochs):
index = np.random.choice(len(df), size=10) # 小批量选取
x_batch = df.iloc[index]['x'].values.reshape(-1, 1)
y_batch = df.iloc[index]['y']
weights = sgd_step(x_batch, y_batch, weights, learning_rate)
print("最终权重:", weights)
```
这个例子中,我们首先生成了一些模拟数据,然后定义了线性回归模型、梯度计算以及SGD更新步骤。每次迭代,我们会随机选择一部分数据(小批量)来进行梯度下降更新。
阅读全文
相关推荐
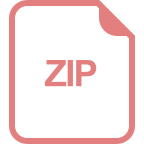
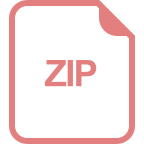


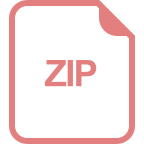
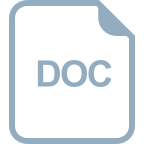
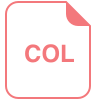
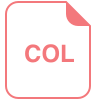
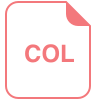
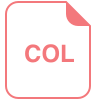
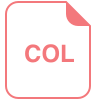
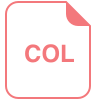
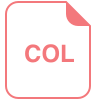
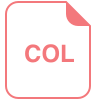
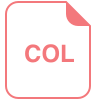
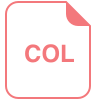
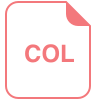
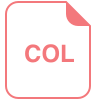