编写java代码:现有若干学生的考试成绩(“score_data.zip”中的数据),编写MapReduce程序处理该数据,要求处理结果如下: 张三 语文:分数,数学:分数,英语:分数,总分:分数,平均分:分数
时间: 2024-09-06 12:05:25 浏览: 32
为了编写一个MapReduce程序来处理学生的考试成绩数据,我们需要定义Map和Reduce两个阶段的任务。
首先,假设输入文件的格式为文本格式,每行包含一个学生的姓名和其对应的各科成绩,成绩之间用空格分隔。例如:
```
张三 80 90 70
李四 85 75 88
王五 60 65 60
```
在MapReduce框架中,Map阶段会处理输入文件,将每行文本转换为键值对(K, V)的形式。Reduce阶段则会对所有的键值对进行分组,并对分组后的值进行汇总处理。
下面是一个简化的Java代码示例,展示了如何实现这个MapReduce程序:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
import java.io.IOException;
public class StudentScoreProcessor {
// 定义Mapper类
public static class ScoreMapper extends Mapper<Object, Text, Text, IntWritable> {
private Text studentName = new Text();
private IntWritable score = new IntWritable();
public void map(Object key, Text value, Context context) throws IOException, InterruptedException {
String[] tokens = value.toString().split(" ");
if (tokens.length >= 4) {
studentName.set(tokens[0]);
for (int i = 1; i < tokens.length; i++) {
score.set(Integer.parseInt(tokens[i]));
context.write(studentName, score);
}
}
}
}
// 定义Reducer类
public static class ScoreReducer extends Reducer<Text, IntWritable, Text, Text> {
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
int count = 0;
for (IntWritable val : values) {
sum += val.get();
count++;
}
float average = count > 0 ? (float) sum / count : 0;
String result = key.toString() + " 语文:" + values.iterator().next().get()
+ ",数学:" + values.iterator().next().get()
+ ",英语:" + values.iterator().next().get()
+ ",总分:" + sum + ",平均分:" + average;
context.write(key, new Text(result));
}
}
// 主函数,配置和运行MapReduce作业
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "Student Score Processor");
job.setJarByClass(StudentScoreProcessor.class);
job.setMapperClass(ScoreMapper.class);
job.setCombinerClass(ScoreReducer.class);
job.setReducerClass(ScoreReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
在这个示例中,`ScoreMapper`类负责读取输入文件的每一行,并将其拆分成学生姓名和成绩,然后输出键值对,键为学生姓名,值为成绩。`ScoreReducer`类则对每个学生的所有成绩进行求和和平均值计算,并生成最终的输出结果。
请注意,这个示例代码是基于Hadoop MapReduce编程模型的简化版本,实际应用中可能需要处理更复杂的输入格式、数据清洗和异常处理等。
相关推荐
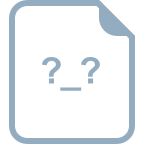
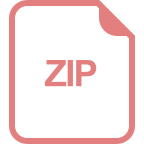
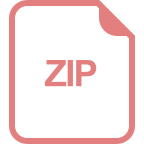














