通过MapReduce框架编写程序统计出每个学生的考试总成绩 用java实现
时间: 2024-02-16 21:00:23 浏览: 79
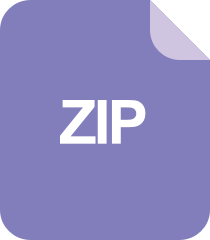
基于Java实现的简易MapReduce框架.zip
好的,我可以帮你解答这个问题。
首先,需要明确一下MapReduce框架的基本原理:将大规模的数据集分成许多小块,分发到多台计算机上进行并行处理,最后将结果汇总起来。
那么,针对你的问题,我们可以采用以下步骤来实现:
1. Map阶段:将每个学生的成绩数据进行处理,将学生姓名作为key,成绩作为value,输出为(key, value)键值对。
2. Reduce阶段:将相同学生的成绩累加起来,得到每个学生的总成绩,最后输出为(key, value)键值对,其中key为学生姓名,value为总成绩。
下面是Java实现代码:
```
public class ScoreMapper extends Mapper<LongWritable, Text, Text, IntWritable> {
@Override
protected void map(LongWritable key, Text value, Context context)
throws IOException, InterruptedException {
String line = value.toString();
String[] fields = line.split(",");
String name = fields[0];
int score = Integer.parseInt(fields[1]);
context.write(new Text(name), new IntWritable(score));
}
}
public class ScoreReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
@Override
protected void reduce(Text key, Iterable<IntWritable> values, Context context)
throws IOException, InterruptedException {
int sum = 0;
for (IntWritable value : values) {
sum += value.get();
}
context.write(key, new IntWritable(sum));
}
}
public class ScoreJob {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "score count");
job.setJarByClass(ScoreJob.class);
job.setMapperClass(ScoreMapper.class);
job.setReducerClass(ScoreReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
代码中,ScoreMapper类继承自Mapper类,实现了map方法,将每个学生的成绩数据转化为(key, value)键值对。ScoreReducer类继承自Reducer类,实现了reduce方法,将相同学生的成绩累加起来,得到每个学生的总成绩。ScoreJob类是MapReduce作业的入口,设置了输入输出路径、Mapper和Reducer类等信息。
你可以将以上代码保存为ScoreMapper.java、ScoreReducer.java和ScoreJob.java三个文件,然后使用hadoop jar命令来执行MapReduce作业,具体命令如下:
```
hadoop jar ScoreJob.jar ScoreJob input output
```
其中,ScoreJob.jar是打包好的Java程序文件,input是输入数据路径,output是输出结果路径。
希望这个回答能够帮助到你!
阅读全文
相关推荐
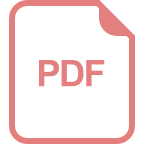















