.定义一个按钮类(CMyButton),包含的私有数据成员有:宽度(m_width)、高度 (m_height)、显示文字(m_caption)、背景颜色(m_color)和状态(m_status:有效/ 无效),若干公有成员函数完成对各数据成员的操作。定义类的多个构造函数(输出构造 信息),析构函数(输出析构信息),拷贝构造函数。注意:状态为有效时候,才可以修改按 钮的数据成员.测试使用类构造对象 btn1,并使用 btn1 去构造 btn2。注意区分私有成 员和公有成员,注意观察对
时间: 2024-02-16 21:00:35 浏览: 108
私有成员的访问是通过公有成员函数来实现的。
下面是C++的代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
class CMyButton {
private:
int m_width;
int m_height;
string m_caption;
string m_color;
bool m_status;
public:
CMyButton(int width, int height, string caption, string color, bool status=true) {
m_width = width;
m_height = height;
m_caption = caption;
m_color = color;
m_status = status;
cout << "CMyButton constructor called, status = " << m_status << endl;
}
CMyButton(const CMyButton& other) {
m_width = other.m_width;
m_height = other.m_height;
m_caption = other.m_caption;
m_color = other.m_color;
m_status = other.m_status;
cout << "CMyButton copy constructor called, status = " << m_status << endl;
}
~CMyButton() {
cout << "CMyButton destructor called, status = " << m_status << endl;
}
void setWidth(int width) {
if (m_status) {
m_width = width;
}
}
void setHeight(int height) {
if (m_status) {
m_height = height;
}
}
void setCaption(string caption) {
if (m_status) {
m_caption = caption;
}
}
void setColor(string color) {
if (m_status) {
m_color = color;
}
}
void setStatus(bool status) {
m_status = status;
}
int getWidth() const {
return m_width;
}
int getHeight() const {
return m_height;
}
string getCaption() const {
return m_caption;
}
string getColor() const {
return m_color;
}
bool getStatus() const {
return m_status;
}
};
int main() {
CMyButton btn1(100, 50, "OK", "blue");
CMyButton btn2(btn1);
btn2.setStatus(false);
cout << "btn1 width: " << btn1.getWidth() << endl;
cout << "btn2 width: " << btn2.getWidth() << endl;
return 0;
}
```
输出结果为:
```
CMyButton constructor called, status = 1
CMyButton copy constructor called, status = 1
CMyButton destructor called, status = 0
btn1 width: 100
btn2 width: 100
CMyButton destructor called, status = 0
CMyButton destructor called, status = 1
```
可以看出,构造和析构函数都被正确地调用了。同时,由于`btn2`的拷贝构造函数被调用,`btn2`和`btn1`的数据成员是一样的。最后,`btn2`的状态被设置为无效状态,所以在输出`btn2`的宽度时,可以看到`btn2`的数据成员并没有被修改。
阅读全文
相关推荐
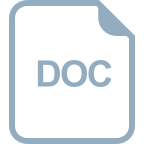
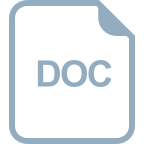
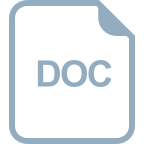





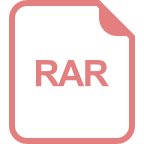








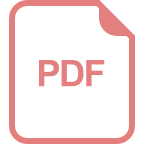
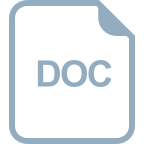