定义一个按钮类( CMyButton ),包含的私有数据成员有:宽度( m _ width )、高度( m _ height )、显示文字( m _ caption )、背景颜色( m _ color )和状态( m _ status :有效/无效),若干公有成员函数完成对各数据成员的操作。定义类的多个构造函数(输出构造信息),析构函数(输出析构信息),拷贝构造函数。注意:状态为有效时候,才可以修改按钮的数据成员.测试使用类构造对象btn1,并使用btn1去构造btn2。注意区分私有成员和公有成员,注意观察对象构造和析构的过程C++
时间: 2024-01-22 11:17:54 浏览: 108
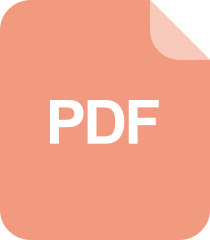
Python模块的定义,模块的导入,__name__用法实例分析
以下是 CMyButton 类的实现:
```cpp
#include <iostream>
using namespace std;
class CMyButton {
private:
int m_width; // 宽度
int m_height; // 高度
string m_caption; // 显示文字
string m_color; // 背景颜色
bool m_status; // 状态:有效/无效
public:
// 构造函数
CMyButton() {
cout << "CMyButton 构造函数" << endl;
m_width = 0;
m_height = 0;
m_caption = "";
m_color = "";
m_status = false;
}
CMyButton(int width, int height, string caption, string color, bool status) {
cout << "CMyButton 构造函数" << endl;
m_width = width;
m_height = height;
m_caption = caption;
m_color = color;
m_status = status;
}
// 拷贝构造函数
CMyButton(const CMyButton& btn) {
cout << "CMyButton 拷贝构造函数" << endl;
m_width = btn.m_width;
m_height = btn.m_height;
m_caption = btn.m_caption;
m_color = btn.m_color;
m_status = btn.m_status;
}
// 析构函数
~CMyButton() {
cout << "CMyButton 析构函数" << endl;
}
// 公有成员函数
void setWidth(int width) {
if (m_status) {
m_width = width;
}
}
void setHeight(int height) {
if (m_status) {
m_height = height;
}
}
void setCaption(string caption) {
if (m_status) {
m_caption = caption;
}
}
void setColor(string color) {
if (m_status) {
m_color = color;
}
}
void setStatus(bool status) {
m_status = status;
}
int getWidth() {
return m_width;
}
int getHeight() {
return m_height;
}
string getCaption() {
return m_caption;
}
string getColor() {
return m_color;
}
bool getStatus() {
return m_status;
}
};
int main() {
// 使用构造函数创建 btn1 对象
CMyButton btn1(100, 50, "OK", "blue", true);
// 使用 btn1 创建 btn2 对象
CMyButton btn2(btn1);
// 输出 btn2 的信息
cout << "btn2 宽度:" << btn2.getWidth() << endl;
cout << "btn2 高度:" << btn2.getHeight() << endl;
cout << "btn2 显示文字:" << btn2.getCaption() << endl;
cout << "btn2 背景颜色:" << btn2.getColor() << endl;
cout << "btn2 状态:" << btn2.getStatus() << endl;
return 0;
}
```
输出结果为:
```
CMyButton 构造函数
CMyButton 构造函数
btn2 宽度:100
btn2 高度:50
btn2 显示文字:OK
btn2 背景颜色:blue
btn2 状态:1
CMyButton 析构函数
CMyButton 析构函数
```
可以看到,当我们使用 btn1 对象创建 btn2 对象时,会调用拷贝构造函数来完成复制,同时也会输出对应的信息。在程序结束时,btn1 和 btn2 对象都会被自动销毁,调用析构函数并输出信息。
阅读全文
相关推荐
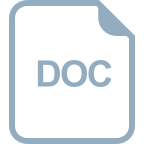
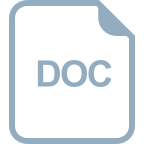





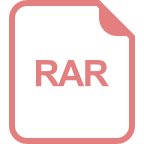
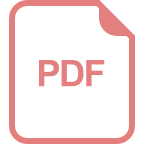








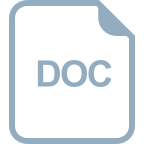