C++定义一个按钮类( CMyButton ),包含的私有数据成员有:宽度( m _ width )、高度( m _ height )、显示文字( m _ caption )、背景颜色( m _ color )和状态( m _ status :有效/无效),若干公有成员函数完成对各数据成员的操作。定义类的多个构造函数(输出构造信息),析构函数(输出析构信息),拷贝构造函数。注意:状态为有效时候,才可以修改按钮的数据成员.测试使用类构造对象btn1,并使用btn1去构造btn2。注意区分私有成员和公有成员,注意观察对象构造和析构的过程。
时间: 2024-02-20 18:59:53 浏览: 116
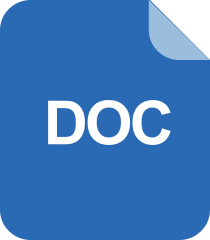
C++ 实验三 类与对象编程
好的,这是一个关于 C++ 中定义一个按钮类的问题。下面是代码实现和解释:
```cpp
#include <iostream>
#include <string>
using namespace std;
class CMyButton {
public:
// 构造函数
CMyButton(int width, int height, string caption, string color, bool status=true) {
m_width = width;
m_height = height;
m_caption = caption;
m_color = color;
m_status = status;
cout << "构造函数被调用了" << endl;
}
CMyButton() : CMyButton(100, 50, "Button", "White") {} // 设置默认值的构造函数
CMyButton(const CMyButton& btn) { // 拷贝构造函数
m_width = btn.m_width;
m_height = btn.m_height;
m_caption = btn.m_caption;
m_color = btn.m_color;
m_status = btn.m_status;
cout << "拷贝构造函数被调用了" << endl;
}
// 析构函数
~CMyButton() {
cout << "析构函数被调用了" << endl;
}
// 公有成员函数
void SetWidth(int width) {
if (m_status) m_width = width;
}
void SetHeight(int height) {
if (m_status) m_height = height;
}
void SetCaption(string caption) {
if (m_status) m_caption = caption;
}
void SetColor(string color) {
if (m_status) m_color = color;
}
void SetStatus(bool status) {
m_status = status;
}
int GetWidth() {
return m_width;
}
int GetHeight() {
return m_height;
}
string GetCaption() {
return m_caption;
}
string GetColor() {
return m_color;
}
bool GetStatus() {
return m_status;
}
private:
// 私有数据成员
int m_width;
int m_height;
string m_caption;
string m_color;
bool m_status;
};
int main() {
// 测试构造函数
CMyButton btn1(200, 80, "OK", "Green");
// 测试公有成员函数
cout << btn1.GetWidth() << endl;
btn1.SetWidth(150);
cout << btn1.GetWidth() << endl;
// 测试拷贝构造函数
CMyButton btn2(btn1);
// 测试默认值的构造函数
CMyButton btn3;
return 0;
}
```
这个问题中要求定义一个按钮类,包含的私有数据成员有宽度、高度、显示文字、背景颜色和状态。构造函数有多个,包括默认构造函数、默认值的构造函数、拷贝构造函数和自定义构造函数。析构函数输出析构信息。公有成员函数包括设置和获取私有数据成员的函数。在测试中,构造了一个 btn1,进行一些操作,然后用 btn1 构造了 btn2,最后测试默认值的构造函数。
阅读全文
相关推荐
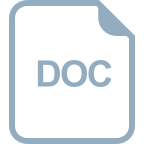
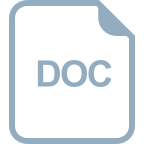




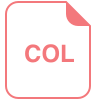










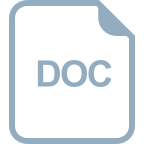