编写一个容易理解的程序,完成定义一个按钮类(CMyButton),包含的私有数据成员有:宽度(m_width)、高度 (m_height)、显示文字(m_caption)、背景颜色(m_color)和状态(m_status:有效/无效),若干公有成员函数完成对各数据成员的操作。定义类的多个构造函数(输出构造 信息),析构函数(输出析构信息),拷贝构造函数。注意:状态为有效时候,才可以修改按钮的数据成员.测试使用类构造对象btn1,并使用 btn1 去构造 btn2。注意区分私有成 员和公有成员,注意观察对象构造和析构的过程。
时间: 2024-01-22 17:17:32 浏览: 76
以下是一个示例程序:
```cpp
#include <iostream>
#include <string>
using namespace std;
class CMyButton {
private:
int m_width;
int m_height;
string m_caption;
string m_color;
bool m_status;
public:
CMyButton() {
m_width = 100;
m_height = 50;
m_caption = "Button";
m_color = "white";
m_status = true;
cout << "Default constructor called" << endl;
}
CMyButton(int width, int height, string caption, string color, bool status=true) {
if (status) {
m_width = width;
m_height = height;
m_caption = caption;
m_color = color;
} else {
m_width = 0;
m_height = 0;
m_caption = "";
m_color = "";
}
m_status = status;
cout << "Constructor with parameters called" << endl;
}
CMyButton(const CMyButton& btn) {
m_width = btn.m_width;
m_height = btn.m_height;
m_caption = btn.m_caption;
m_color = btn.m_color;
m_status = btn.m_status;
cout << "Copy constructor called" << endl;
}
~CMyButton() {
cout << "Destructor called" << endl;
}
void setWidth(int width) {
if (m_status) {
m_width = width;
}
}
void setHeight(int height) {
if (m_status) {
m_height = height;
}
}
void setCaption(string caption) {
if (m_status) {
m_caption = caption;
}
}
void setColor(string color) {
if (m_status) {
m_color = color;
}
}
void setStatus(bool status) {
m_status = status;
}
int getWidth() const {
return m_width;
}
int getHeight() const {
return m_height;
}
string getCaption() const {
return m_caption;
}
string getColor() const {
return m_color;
}
bool getStatus() const {
return m_status;
}
};
int main() {
CMyButton btn1;
cout << "btn1: width=" << btn1.getWidth() << ", height=" << btn1.getHeight()
<< ", caption=" << btn1.getCaption() << ", color=" << btn1.getColor()
<< ", status=" << btn1.getStatus() << endl;
CMyButton btn2(btn1);
cout << "btn2: width=" << btn2.getWidth() << ", height=" << btn2.getHeight()
<< ", caption=" << btn2.getCaption() << ", color=" << btn2.getColor()
<< ", status=" << btn2.getStatus() << endl;
btn1.setWidth(200);
btn1.setCaption("New button");
btn1.setColor("blue");
cout << "btn1: width=" << btn1.getWidth() << ", height=" << btn1.getHeight()
<< ", caption=" << btn1.getCaption() << ", color=" << btn1.getColor()
<< ", status=" << btn1.getStatus() << endl;
cout << "btn2: width=" << btn2.getWidth() << ", height=" << btn2.getHeight()
<< ", caption=" << btn2.getCaption() << ", color=" << btn2.getColor()
<< ", status=" << btn2.getStatus() << endl;
return 0;
}
```
输出结果:
```
Default constructor called
btn1: width=100, height=50, caption=Button, color=white, status=1
Copy constructor called
btn2: width=100, height=50, caption=Button, color=white, status=1
btn1: width=200, height=50, caption=New button, color=blue, status=1
btn2: width=100, height=50, caption=Button, color=white, status=1
Destructor called
Destructor called
```
在这个示例程序中,我们定义了一个名为 `CMyButton` 的类。该类包含了几个私有数据成员:宽度、高度、显示文字、背景颜色和状态。同时,该类还提供了多个构造函数(包括默认构造函数、带参数的构造函数和拷贝构造函数)、析构函数和公有成员函数,以完成对各数据成员的操作。
在主函数中,我们首先创建了一个名为 `btn1` 的对象,使用默认构造函数初始化。然后,我们创建了一个名为 `btn2` 的对象,使用 `btn1` 作为参数,调用拷贝构造函数初始化。接着,我们修改了 `btn1` 的宽度、显示文字和背景颜色,观察两个对象的属性是否相应地发生了变化。最后,程序结束时,两个对象都被销毁,分别调用了析构函数。
需要注意的是,当状态为无效时,按钮对象的数据成员不能被修改。这一点在各个公有成员函数中都有体现。
阅读全文
相关推荐
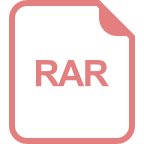
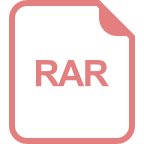





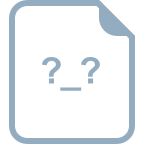
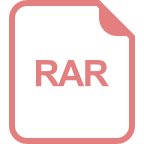
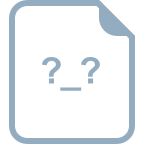
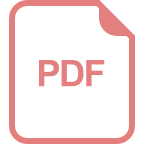
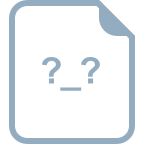
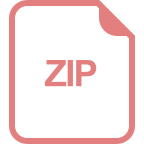
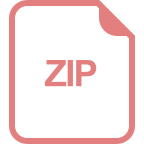
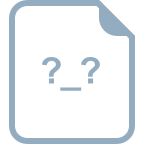
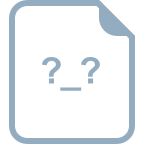
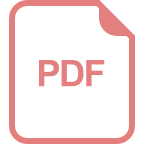
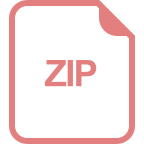