遗传算法python编码例子
时间: 2023-12-20 13:05:18 浏览: 34
遗传算法的编码方式有多种,其中二进制编码是最常用的一种。下面是一个使用二进制编码的遗传算法Python示例:
```python
import random
# 定义目标函数
def fitness_func(chromosome):
x = decode(chromosome[:10], -5, 5)
y = decode(chromosome[10:], -5, 5)
return x**2 + y**2
# 定义解码函数
def decode(binary, a, b):
x = int(''.join(str(i) for i in binary), 2)
return a + (b - a) * x / (2**len(binary) - 1)
# 定义交叉函数
def crossover(parent1, parent2):
point = random.randint(1, len(parent1) - 1)
child1 = parent1[:point] + parent2[point:]
child2 = parent2[:point] + parent1[point:]
return child1, child2
# 定义变异函数
def mutation(chromosome, p):
for i in range(len(chromosome)):
if random.random() < p:
chromosome[i] = 1 - chromosome[i]
return chromosome
# 初始化种群
population_size = 50
chromosome_length = 20
population = [[random.randint(0, 1) for j in range(chromosome_length)] for i in range(population_size)]
# 开始迭代
max_generation = 100
crossover_rate = 0.8
mutation_rate = 0.01
for generation in range(max_generation):
# 计算适应度
fitness = [fitness_func(chromosome) for chromosome in population]
# 选择
parents = []
for i in range(population_size):
parent1 = population[roulette_wheel_selection(fitness)]
parent2 = population[roulette_wheel_selection(fitness)]
parents.append((parent1, parent2))
# 交叉
offspring = []
for parent1, parent2 in parents:
if random.random() < crossover_rate:
child1, child2 = crossover(parent1, parent2)
offspring.append(child1)
offspring.append(child2)
else:
offspring.append(parent1)
offspring.append(parent2)
# 变异
population = [mutation(chromosome, mutation_rate) for chromosome in offspring]
# 输出最优解
best_chromosome = population[0]
best_fitness = fitness_func(best_chromosome)
for chromosome in population:
fitness = fitness_func(chromosome)
if fitness < best_fitness:
best_chromosome = chromosome
best_fitness = fitness
x = decode(best_chromosome[:10], -5, 5)
y = decode(best_chromosome[10:], -5, 5)
print("x =", x)
print("y =", y)
print("f(x,y) =", best_fitness)
```
在这个例子中,我们使用二进制编码来表示自变量x和y。每个染色体由20个基因组成,前10个基因表示x,后10个基因表示y。解码函数decode将二进制编码转换为实数值。交叉函数crossover和变异函数mutation用于产生新的染色体。选择函数roulette_wheel_selection使用轮盘赌算法来选择父代。最终,我们得到了最优解x、y和目标函数的最小值f(x,y)。
相关推荐
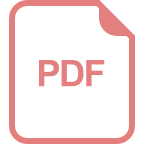














