对于stm32f103c6的LCD1602的头文件是什么
时间: 2024-03-07 11:50:16 浏览: 23
对于stm32f103c6驱动LCD1602液晶屏,需要使用以下头文件:
```
#include "stm32f10x.h"
#include "delay.h"
#include "lcd1602.h"
```
其中,"stm32f10x.h"是STM32的标准头文件,"delay.h"是延时函数的头文件,"lcd1602.h"是LCD1602液晶屏的头文件。你需要在代码中先定义这些头文件,才能使用对应的函数和宏定义。
相关问题
编写stm32f103c6t6用LCD1602显示数字的代码
以下是基于STM32CubeIDE的示例代码,可以在STM32F103C6T6微控制器上使用LCD1602显示数字:
```c
#include "main.h"
#include "lcd1602.h"
int main(void)
{
HAL_Init();
SystemClock_Config();
// 初始化LCD1602
lcd1602_init();
uint8_t num = 0;
char num_str[4];
while (1)
{
// 将数字转换为字符串
sprintf(num_str, "%d", num);
// 在第一行第一列显示数字
lcd1602_set_cursor(0, 0);
lcd1602_send_string(num_str);
HAL_Delay(1000); // 延时1秒
num++;
}
}
```
需要在项目中添加lcd1602.c和lcd1602.h文件,并在main.c中包含lcd1602.h头文件。在lcd1602.c文件中,需要根据具体的硬件连接情况修改GPIO引脚和时钟等相关设置。
对于stm32f103c6的LCD1602的文件加进去会在stm32f10x.h这个文件里面出现很多错误
如果在包含了 `lcd1602.h` 头文件后,编译时出现很多错误提示,可能是因为 `lcd1602.h` 文件中定义了一些与 `stm32f10x.h` 中的定义冲突的宏或类型,导致编译器无法识别或处理这些定义。你可以尝试一下以下几个方法,看看是否能够解决这个问题:
1. 确认头文件包含顺序:在包含 `lcd1602.h` 头文件之前,先包含 `stm32f10x.h` 头文件,这样可以避免一些定义冲突的问题。
2. 检查宏定义:如果 `lcd1602.h` 中定义了与 `stm32f10x.h` 中的宏定义冲突的宏,可以尝试修改 `lcd1602.h` 中的宏定义,或者在包含 `lcd1602.h` 头文件之前,先包含一个空的头文件,例如:
```
#include "stm32f10x.h"
#include "empty.h"
#include "lcd1602.h"
```
这样可以避免宏定义冲突的问题。
3. 检查类型定义:如果 `lcd1602.h` 中定义了与 `stm32f10x.h` 中的类型定义冲突的类型,可以尝试修改 `lcd1602.h` 中的类型定义,或者在包含 `lcd1602.h` 头文件之前,先包含一个空的头文件,例如:
```
#include "stm32f10x.h"
#include "empty.h"
#include "lcd1602.h"
```
这样可以避免类型定义冲突的问题。
希望以上方法能够帮到你解决问题。如果还有问题,请提供具体的错误提示信息和代码,方便我更好地帮助你。
相关推荐
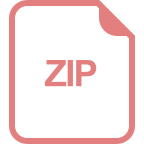
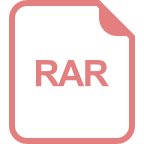









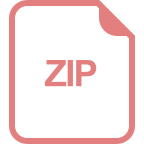
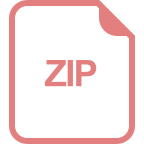
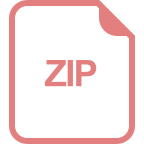