stm32串口发送1控制led亮
时间: 2024-09-20 21:12:26 浏览: 63
STM32单片机的串口通信通常用于将数据传输到其他设备,如PC或者其他的微控制器。如果你想通过串口控制LED灯,你需要做以下几个步骤:
1. **配置串口**:首先,在STM32中,启用并配置USART(通用异步收发器),设置波特率、数据位数、停止位以及校验模式等。
```c
void USART_Init(void) {
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
//...初始化GPIO用于连接LED和TX/RX pins...
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB1Periph_USART2, ENABLE);
//...配置TX和RX GPIO...
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9; //假设TX为PA9
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP; //推挽输出
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 9600; //设置波特率为9600bps
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx | USART_Mode_Rx;
USART_Init(USART2, &USART_InitStructure);
USART_Cmd(USART2, ENABLE); //启动串口
}
```
2. **发送数据**:当你想要点亮LED时,需要发送特定的数据字符(比如0x01表示亮)。你可以创建一个函数来处理这个过程:
```c
void SendLedCommand(uint8_t ledState) {
uint8_t data = ledState << 4; //如果LED状态为1,左移一位作为高四位
USART_SendData(USART2, data); //发送数据到串口
}
```
3. **接收反馈**:虽然大部分时候LED控制不需要接收反馈,但在某些复杂系统中,你可能希望确认数据是否正确到达了目标设备。这就涉及到添加错误检测机制。
4. **LED控制循环**:最后,在主程序中定期调用`SendLedCommand`函数来控制LED的状态。例如:
```c
while (1) {
if (/* 某个条件触发 */)
SendLedCommand(LED_ON); // LED亮
else
SendLedCommand(LED_OFF); // LED灭
delay_ms(500); // 等待一段时间再改变状态
}
```
相关推荐
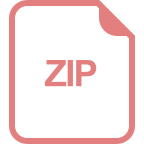
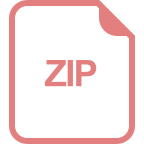
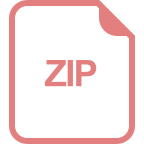













