基于STM32的串口控制LED亮灭
时间: 2023-07-26 21:43:14 浏览: 138
好的,我可以为你提供相应的代码,但在此之前,需要确认一下你使用的是哪个型号的STM32芯片以及LED是如何连接的。同时,你需要先了解一下串口通信的相关知识,比如波特率、数据位、停止位、奇偶校验等。这些都是在代码中需要配置的参数。
假设你使用的是STM32F103C8T6芯片,并且LED连接在该芯片的PB12引脚上,可以参考以下代码实现串口控制LED亮灭:
```c
#include "stm32f10x.h"
void USART1_Config(void);
void LED_Config(void);
int main(void)
{
USART1_Config(); // 配置串口1
LED_Config(); // 配置LED引脚
while (1)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET); // 等待接收到数据
uint8_t data = USART_ReceiveData(USART1); // 接收数据
if (data == '1')
{
GPIO_SetBits(GPIOB, GPIO_Pin_12); // 点亮LED
}
else if (data == '0')
{
GPIO_ResetBits(GPIOB, GPIO_Pin_12); // 熄灭LED
}
}
}
// 配置串口1,波特率为9600,数据位为8,停止位为1,无奇偶校验
void USART1_Config(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
// 配置USART1的TX引脚
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1的RX引脚
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1的参数
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
// 配置LED引脚
void LED_Config(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_12;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
```
在这个示例代码中,我们使用了USART1进行串口通信,波特率为9600,数据位为8,停止位为1,无奇偶校验。当接收到的数据为字符'1'时,点亮LED;当接收到的数据为字符'0'时,熄灭LED。你可以在串口调试助手中发送相应的字符,来控制LED的亮灭。
当然,这只是一个简单的示例,具体的代码实现还需要根据你的具体需求进行适当的修改。
阅读全文
相关推荐
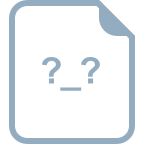
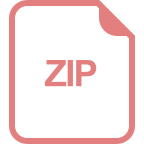
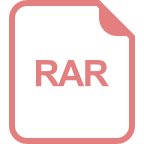
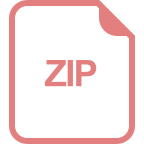
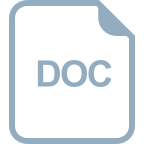
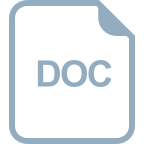

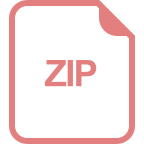
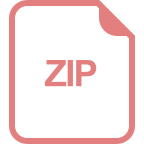
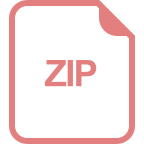
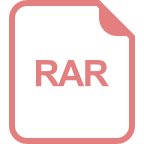
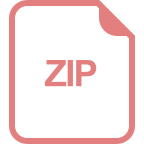
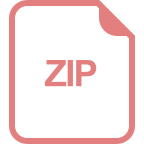
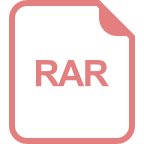
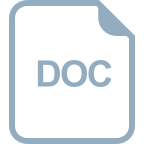
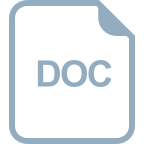
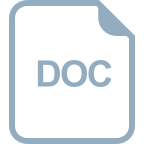
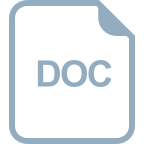