C++实现前缀 中缀 后缀的相互转换代码
时间: 2024-11-13 22:18:56 浏览: 4
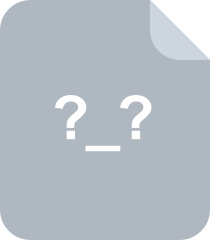
简易计算器 C++实现 基于栈 中缀转后缀
C++中,我们可以使用栈数据结构来实现表达式的前缀、中缀和后缀之间的转换。这里给出简单的代码示例:
**前缀到后缀的转换(Shunting Yard算法):**
```cpp
#include <iostream>
#include <stack>
#include <string>
#include <cctype>
std::string prefixToPostfix(const std::string& prefix) {
std::stack<char> opStack;
std::string postfix = "";
for (char c : prefix) {
if (isdigit(c)) {
postfix += c; // 直接添加数字
} else if (c == '(' || isalpha(c)) { // 遇到操作符或左括号,压入堆栈
opStack.push(c);
} else if (c == ')') { // 遇到右括号,弹出并添加直到遇到左括号
while (!opStack.empty() && opStack.top() != '(') {
postfix += opStack.top();
opStack.pop();
}
if (!opStack.empty()) {
opStack.pop(); // 弹出左括号
}
} else { // 遇到运算符,处理优先级较高的先入栈
while (!opStack.empty() && precedence(c) <= precedence(opStack.top())) {
postfix += opStack.top();
opStack.pop();
}
opStack.push(c);
}
}
// 最后将剩余的操作符加入后缀表达式
while (!opStack.empty()) {
postfix += opStack.top();
opStack.pop();
}
return postfix;
}
// 运算符优先级函数,可以根据实际需求调整
int precedence(char op) {
switch (op) {
case '+': case '-':
case '*': case '/':
return 1;
default:
return 0;
}
}
int main() {
std::string prefixExpr = "3+5*2-1";
std::string postfixExpr = prefixToPostfix(prefixExpr);
std::cout << "Prefix to Postfix: " << postfixExpr << "\n";
return 0;
}
```
**后缀到前缀的转换:**
```cpp
bool isOperator(const char& ch) {
return (ch >= 'A' && ch <= 'Z') || (ch >= 'a' && ch <= 'z');
}
std::string postfixToPrefix(const std::string& postfix) {
std::stack<std::pair<char, int>> stack;
for (char c : postfix) {
if (isdigit(c)) {
stack.push({c - '0', 1}); // 保持数字不变
} else if (isOperator(c)) {
while (!stack.empty() && !isOperator(stack.top().first) && precedence(stack.top().first) >= precedence(c)) {
postfix.insert(postfix.begin(), stack.top().first);
stack.pop();
}
stack.push({c, 1});
} else if (c == '(') {
stack.push({c, 0});
} else if (c == ')') {
while (stack.top().second != 0) {
postfix.insert(postfix.begin(), stack.top().first);
stack.pop();
}
stack.pop(); // 弹出左括号
}
}
while (!stack.empty()) {
postfix.insert(postfix.begin(), stack.top().first);
stack.pop();
}
return postfix;
}
int main() {
std::string postfixExpr = "34+56*2-1/";
std::string prefixExpr = postfixToPrefix(postfixExpr);
std::cout << "Postfix to Prefix: " << prefixExpr << "\n";
return 0;
}
```
阅读全文
相关推荐
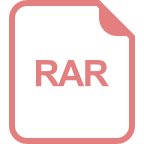
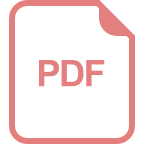


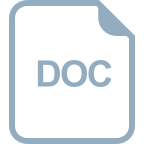




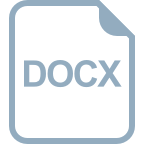






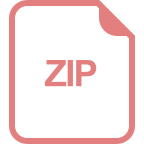
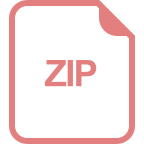
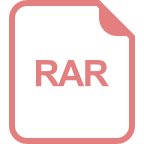