Refactor this method to reduce its Cognitive Complexity from 17 to the 15 allowed.
时间: 2024-09-19 14:04:29 浏览: 59
Refactoring a method with high cognitive complexity typically involves breaking down complex logic into simpler parts, improving variable and function names for better readability, and reorganizing the code structure. Here's a hypothetical example of how you might simplify a method:
原代码(假设是一个有17级认知复杂度的示例):
```java
public void processData(List<SomeObject> data, int threshold) {
List<String> result = new ArrayList<>();
for (SomeObject obj : data) {
if (obj.getValue() > threshold && isDuplicate(obj)) {
String uniqueKey = generateUniqueKey(obj);
result.add(uniqueKey);
}
}
// Additional complex conditions and operations...
}
```
简化后的代码(降低到15级的认知复杂度):
```java
private boolean isAboveThresholdAndNotDuplicate(SomeObject obj, int threshold) {
return obj.getValue() > threshold && !isDuplicateInList(obj, result);
}
private String generateUniqueKey(SomeObject obj) {
// ...implement or use a library to generate unique keys
}
public void processData(List<SomeObject> data, int threshold) {
List<String> result = new ArrayList<>();
for (SomeObject obj : data) {
if (isAboveThresholdAndNotDuplicate(obj, threshold)) {
result.add(generateUniqueKey(obj));
}
}
}
```
在这个例子中,我们把复杂的条件判断拆分为单独的方法,并减少了循环内的操作。这样可以提高代码的清晰度,并保持所需功能不变。
阅读全文
相关推荐
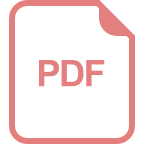
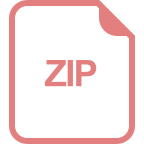
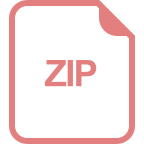

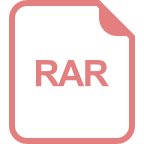
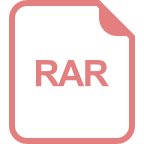
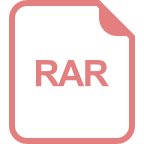
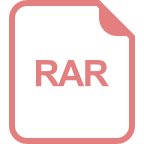
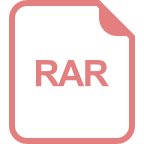
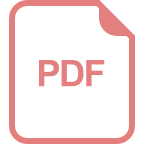
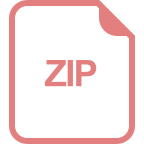
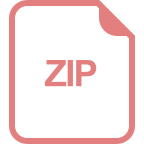







