Refactor the Code Population Generator In the lecture, we created the Animal class for FoxesAndRabbits. If you are looking at this coursework before we had this lecture, you can compare the code in the Coursework repository with the code in the Live Sessions repository. Also, note the changes made to the simulateOneStep method in Simulator.java: By introducing the Animal superclass, we have removed the dependencies (couplings) of the simulateOneStep method and the Fox and Rabbit class. The Simulator class, however, is still coupled to Fox and Rabbit in the populate method. Your task is to further improve FoxesAndRabbits by introducing a new class PopulationGenerator and move the populate method from the Simulator.java into this new class. You might also have to move some of the variables and fix the resulting errors in other classes to now use the PopulationGenerator instead. Now only the PopulationGenerator should be coupled to the concrete animal classes (Fox and Rabbit), making it easier to find places where changes are necessary when the application is extended. The PopulationGenerator should be called in Simulator.java. (Created new class: 0.5P, Moved method to new class: 0.5P, Simulator is using new class without errors: 1P) Introduce New Animals Define a new type of animal
时间: 2024-02-05 15:03:12 浏览: 135
Thank you for your question! Here's an example of how the PopulationGenerator class could be implemented:
```java
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class PopulationGenerator {
private final int width;
private final int height;
private final Random random;
private final List<Animal> animals;
public PopulationGenerator(int width, int height) {
this.width = width;
this.height = height;
this.random = new Random();
this.animals = new ArrayList<>();
}
public void populate(int foxes, int rabbits, int newAnimalType) {
for (int i = 0; i < foxes; i++) {
Location location = new Location(random.nextInt(width), random.nextInt(height));
Fox fox = new Fox(true, location);
animals.add(fox);
}
for (int i = 0; i < rabbits; i++) {
Location location = new Location(random.nextInt(width), random.nextInt(height));
Rabbit rabbit = new Rabbit(true, location);
animals.add(rabbit);
}
for (int i = 0; i < newAnimalType; i++) {
Location location = new Location(random.nextInt(width), random.nextInt(height));
NewAnimal newAnimal = new NewAnimal(true, location);
animals.add(newAnimal);
}
}
public List<Animal> getAnimals() {
return animals;
}
}
```
This class takes in the width and height of the field as constructor arguments and has a `populate` method that creates a specified number of Foxes, Rabbits, and a new type of animal called `NewAnimal`. The `NewAnimal` class would need to be implemented separately with its own behavior.
To use this class in the Simulator, we can create an instance of `PopulationGenerator` and call its `populate` method with the appropriate arguments:
```java
PopulationGenerator populationGenerator = new PopulationGenerator(FIELD_WIDTH, FIELD_HEIGHT);
populationGenerator.populate(NUM_FOXES, NUM_RABBITS, NUM_NEW_ANIMALS);
List<Animal> animals = populationGenerator.getAnimals();
Simulator simulator = new Simulator(FIELD_WIDTH, FIELD_HEIGHT, animals);
```
This way, the Simulator class no longer needs to know about the specific animal classes being used, only the `Animal` superclass.
阅读全文
相关推荐
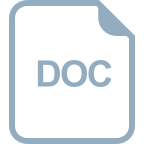
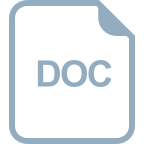
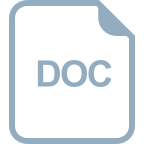
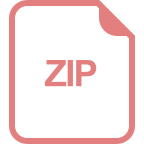
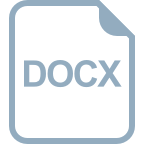
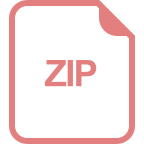
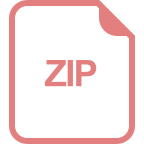
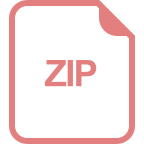
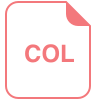







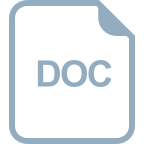
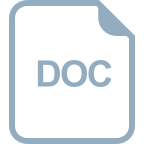
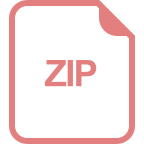