用stm32f103c8t6实现平衡车的pid控制代码
时间: 2023-07-29 13:07:41 浏览: 81
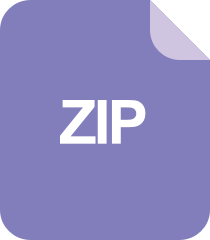
基于stm32的平衡车代码
实现平衡车的PID控制代码需要以下几个步骤:
1. 读取平衡车的姿态角度。
2. 计算平衡车的偏差值。
3. 根据偏差值计算PID控制量。
4. 根据PID控制量控制平衡车的速度。
以下是一个使用stm32f103c8t6的平衡车PID控制代码的示例:
```c
#include "stm32f10x.h"
// 定义PID参数
float kp = 1.0;
float ki = 0.1;
float kd = 0.01;
// 定义计数器
uint32_t count = 0;
// 定义姿态角度
float angle = 0;
// 定义偏差值
float error = 0;
float last_error = 0;
float integral = 0;
float derivative = 0;
// 定义速度控制量
float control = 0;
int main(void)
{
// 初始化GPIO、USART等外设
// ...
while(1)
{
// 读取姿态角度
angle = read_angle();
// 计算偏差值
error = angle - 0;
// 计算PID控制量
integral += error;
derivative = error - last_error;
control = kp * error + ki * integral + kd * derivative;
last_error = error;
// 控制速度
set_speed(control);
// 计数器加1
count++;
if(count >= 1000)
{
// 每秒输出一次偏差值和控制量
printf("error: %f, control: %f\r\n", error, control);
count = 0;
}
}
}
```
在这个例子中,我们假设平衡车的目标角度为0度。首先,我们读取平衡车的实际姿态角度,然后计算出偏差值。接下来,我们使用PID控制算法计算出控制量,最后根据控制量控制平衡车的速度。
请注意,这只是一个示例代码,实际的平衡车PID控制代码需要根据具体的硬件和控制要求进行修改和优化。
阅读全文
相关推荐
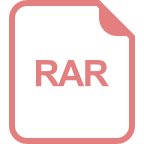


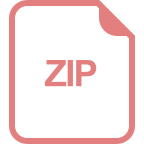
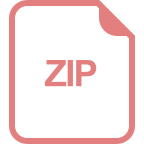
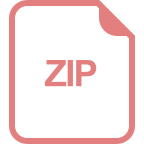
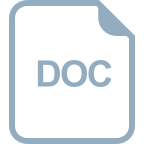
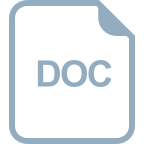
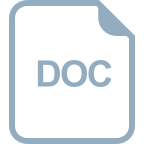




