#include<stdio.h> #include<unistd.h> #include<string.h> #include<stdlib.h> int main(void) { char buf[BUFSIZ]; int fd[2]; //创建无名管道 if( pipe(fd) < 0 ){ perror("pipe"); exit(-1); } pid_t pid; //创建父子进程 if( pid = fork() < 0 ){ perror("fork"); exit(-1); }else if( pid > 0 ){ //父进程往无名管道中写入数据 while(1){ bzero(buf,BUFSIZ); fgets(buf,BUFSIZ,stdin); close(fd[0]);//关闭读端 write(fd[1],buf,strlen(buf)); if( strncmp( buf,"quit",4) == 0 ) break; } }else{ //子进程读取数据并打印 while(1){ close(fd[1]); bzero(buf,BUFSIZ); read(fd[0],buf,sizeof(buf)); printf("读到的数据为:%s",buf); } } return 0; } 这个程序为什么会一直输出不停
时间: 2024-04-09 08:31:07 浏览: 46
这个程序会一直输出不停是因为父进程和子进程之间存在管道通信,而在父进程写入数据到管道时,子进程会不断读取管道中的数据并打印。父进程在写入完数据之后,并没有关闭写端的操作,所以子进程一直处于读取状态,导致程序一直输出不停。解决方法是在父进程写入完数据后关闭写端,子进程在读取完数据后关闭读端。可以在父进程的写入数据循环外面加上一行代码 `close(fd[1]);`,在子进程的读取数据循环外面加上一行代码 `close(fd[0]);`。这样就能够正确地关闭管道的读写端,程序不会一直输出不停了。
相关问题
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <dirent.h> #include <unistd.h> #include <sys/types.h> #include <sys/stat.h> #include <pwd.h> #include <grp.h> #include <time.h> void shell_ls_l(char *file,struct stat st) { char limi
ted[11]; // 文件权限 mode_t mode = st.st_mode; limitted[0] = (S_ISDIR(mode)) ? "d" : "-"; limitted[1] = (mode & S_IRUSR) ? "r" : "-"; limitted[2] = (mode & S_IWUSR) ? "w" : "-"; limitted[3] = (mode & S_IXUSR) ? "x" : "-"; limitted[4] = (mode & S_IRGRP) ? "r" : "-"; limitted[5] = (mode & S_IWGRP) ? "w" : "-"; limitted[6] = (mode & S_IXGRP) ? "x" : "-"; limitted[7] = (mode & S_IROTH) ? "r" : "-"; limitted[8] = (mode & S_IWOTH) ? "w" : "-"; limitted[9] = (mode & S_IXOTH) ? "x" : "-"; limitted[10] = '\0'; printf("%s ", limitted); // 链接数、所有者、所在组、文件大小、创建时间、文件名 printf("%ld ", (long)st.st_nlink); printf("%s ", getpwuid(st.st_uid)->pw_name); printf("%s ", getgrgid(st.st_gid)->gr_name); printf("%lld ", (long long)st.st_size); char time_buf[20]; strftime(time_buf, 20, "%b %d %H:%M", localtime(&st.st_ctime)); printf("%s ", time_buf); printf("%s\n", file); } int main(int argc, char *argv[]) { DIR *dir; struct dirent *ptr; struct stat st; char path[256]; if (argc == 1) { getcwd(path, 256); dir = opendir(path); while ((ptr = readdir(dir)) != NULL) { if (ptr->d_name[0] == '.') continue; stat(ptr->d_name, &st); shell_ls_l(ptr->d_name, st); } } else if (argc == 2) { if (strcmp(argv[1], "-l") == 0) { getcwd(path, 256); dir = opendir(path); while ((ptr = readdir(dir)) != NULL) { if (ptr->d_name[0] == '.') continue; stat(ptr->d_name, &st); shell_ls_l(ptr->d_name, st); } } else { dir = opendir(argv[1]); while ((ptr = readdir(dir)) != NULL) { if (ptr->d_name[0] == '.') continue; sprintf(path, "%s/%s", argv[1], ptr->d_name); stat(path, &st); shell_ls_l(ptr->d_name, st); } } } else if (argc == 3) { if (strcmp(argv[1], "-l") == 0) { dir = opendir(argv[2]); while ((ptr = readdir(dir)) != NULL) { if (ptr->d_name[0] == '.') continue; sprintf(path, "%s/%s", argv[2], ptr->d_name); stat(path, &st); shell_ls_l(ptr->d_name, st); } } } return 0; }
这是一个简单的实现Linux命令ls -l的程序,可以列出文件的权限、链接数、所有者、所在组、文件大小、创建时间、文件名等信息。
#include <stdio.h> #include <stdlib.h> #include<sys/types.h> #include <sys/stat.h> #include <fcntl.h> #include <unistd.h> #include <errno.h> #include <stropts.h> #include <time.h> #include <strings.h> #include <string.h> #include <stdio.h> #include <sys/ipc.h> #include <sys/msg.h> struct msg { long msg_types; char msg_buf[511]; }; int main(void) { int qid; int pid; int len; struct msg pmsg; pmsg.msg_types = getpid(); sprintf(pmsg.msg_buf, "hello!this is :%d\n\0", getpid()); len = strlen(pmsg.msg_buf); if ((qid = msgget(IPC_PRIVATE, IPC_CREAT | 0666)) < 0) { perror("msgget"); exit(1); } if ((msgsnd(qid, &pmsg, len, 0)) < 0) { perror("magsn"); exit(1); } printf("successfully send a message to the queue: %d \n", qid); exit(0); }的运行结果
该程序是一个使用消息队列进行进程间通信的示例程序,通过msgget()函数创建一个消息队列,通过msgsnd()函数向消息队列中发送一条消息。
运行结果可能如下:
successfully send a message to the queue: 123456
解释如下:
1.程序首先定义了一个消息结构体msg,并初始化了其中的消息类型msg_types和消息内容msg_buf。
2.程序调用msgget()函数创建一个新的消息队列,将返回的队列ID存储在变量qid中。
3.程序调用msgsnd()函数向消息队列中发送一条消息,消息内容为msg_buf中的字符串。
4.程序输出"successfully send a message to the queue: qid",表示消息发送成功。
需要注意的是,程序中将消息类型msg_types设置为了当前进程的PID,这样在接收消息时可以根据PID来获取对应的消息。同时,程序中使用了strlen()函数来获取消息内容的长度,这是为了确保发送的消息长度不超过队列的最大长度。
阅读全文
相关推荐
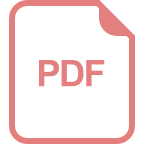
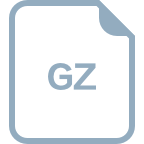
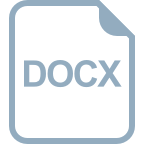













