stm32从小到大输出pwm
时间: 2023-11-12 08:07:16 浏览: 36
STM32是一款32位的微控制器,支持多种输出功能,包括PWM输出。下面是一些从小到大输出PWM的示例代码:
1. 通过直接操作寄存器来输出PWM
```c
#include "stm32f10x.h"
int main(void)
{
uint16_t duty_cycle = 0;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStruct);
TIM_TimeBaseInitTypeDef TIM_InitStruct;
TIM_InitStruct.TIM_Prescaler = 7200 - 1; // 时钟预分频
TIM_InitStruct.TIM_Period = 2000 - 1; // 计数器周期
TIM_InitStruct.TIM_ClockDivision = TIM_CKD_DIV1; // 时钟分割
TIM_InitStruct.TIM_CounterMode = TIM_CounterMode_Up; // 计数器模式
TIM_TimeBaseInit(TIM2, &TIM_InitStruct);
TIM_OCInitTypeDef TIM_OC_InitStruct;
TIM_OC_InitStruct.TIM_OCMode = TIM_OCMode_PWM1; // PWM模式1
TIM_OC_InitStruct.TIM_OutputState = TIM_OutputState_Enable; // 输出使能
TIM_OC_InitStruct.TIM_Pulse = duty_cycle; // 占空比
TIM_OC_InitStruct.TIM_OCPolarity = TIM_OCPolarity_High; // 输出极性
TIM_OC1Init(TIM2, &TIM_OC_InitStruct);
TIM_Cmd(TIM2, ENABLE); // 开启计数器
while (1)
{
for (duty_cycle = 0; duty_cycle <= 2000; duty_cycle += 100)
{
TIM_SetCompare1(TIM2, duty_cycle); // 更新占空比
delay(100); // 延时
}
}
}
```
2. 利用库函数来输出PWM
```c
#include "stm32f10x.h"
int main(void)
{
uint16_t duty_cycle = 0;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStruct);
TIM_TimeBaseInitTypeDef TIM_InitStruct;
TIM_InitStruct.TIM_Prescaler = 7200 - 1; // 时钟预分频
TIM_InitStruct.TIM_Period = 2000 - 1; // 计数器周期
TIM_InitStruct.TIM_ClockDivision = TIM_CKD_DIV1; // 时钟分割
TIM_InitStruct.TIM_CounterMode = TIM_CounterMode_Up; // 计数器模式
TIM_TimeBaseInit(TIM2, &TIM_InitStruct);
TIM_OCInitTypeDef TIM_OC_InitStruct;
TIM_OC_InitStruct.TIM_OCMode = TIM_OCMode_PWM1; // PWM模式1
TIM_OC_InitStruct.TIM_OutputState = TIM_OutputState_Enable; // 输出使能
TIM_OC_InitStruct.TIM_Pulse = duty_cycle; // 占空比
TIM_OC_InitStruct.TIM_OCPolarity = TIM_OCPolarity_High; // 输出极性
TIM_OC1Init(TIM2, &TIM_OC_InitStruct);
TIM_Cmd(TIM2, ENABLE); // 开启计数器
while (1)
{
for (duty_cycle = 0; duty_cycle <= 2000; duty_cycle += 100)
{
TIM_OC1Init(TIM2, &TIM_OC_InitStruct); // 更新占空比
delay(100); // 延时
}
}
}
```
以上两段代码均可实现从小到大输出PWM的功能。其中,第一段代码直接操作寄存器来配置PWM输出,第二段代码利用了库函数来简化操作。
相关推荐
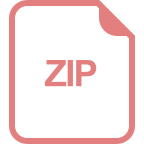
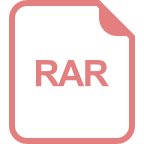
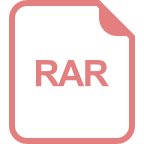














