yolov5实时检测屏幕并输出坐标python代码
时间: 2023-07-21 14:28:51 浏览: 75
以下是使用 YOLOv5 实时检测屏幕并输出检测框的左上角和右下角坐标的 Python 代码示例:
```python
import cv2
import numpy as np
import time
from PIL import ImageGrab
# 加载 YOLOv5 检测模型
net = cv2.dnn.readNet("yolov5.weights", "yolov5.cfg")
classes = []
with open("coco.names", "r") as f:
classes = [line.strip() for line in f.readlines()]
# 设置输入图片大小
input_size = (640, 640)
# 循环检测屏幕
while True:
start_time = time.time()
# 获取屏幕截图
img = ImageGrab.grab(bbox=(0, 0, 1920, 1080))
# 将 PIL 图片转换为 OpenCV 图片
img = cv2.cvtColor(np.array(img), cv2.COLOR_RGB2BGR)
# 对图片进行预处理
img = cv2.resize(img, input_size)
img = img.transpose((2, 0, 1))
img = np.expand_dims(img, axis=0)
img = img / 255.0
# 运行 YOLOv5 检测
net.setInput(img)
outputs = net.forward()
# 处理检测结果
conf_threshold = 0.5
nms_threshold = 0.4
boxes = []
confidences = []
class_ids = []
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > conf_threshold:
center_x = int(detection[0] * input_size[0])
center_y = int(detection[1] * input_size[1])
width = int(detection[2] * input_size[0])
height = int(detection[3] * input_size[1])
left = int(center_x - width / 2)
top = int(center_y - height / 2)
boxes.append([left, top, width, height])
confidences.append(float(confidence))
class_ids.append(class_id)
indices = cv2.dnn.NMSBoxes(boxes, confidences, conf_threshold, nms_threshold)
for i in indices:
i = i[0]
box = boxes[i]
left = box[0]
top = box[1]
width = box[2]
height = box[3]
label = classes[class_ids[i]]
cv2.rectangle(img, (left, top), (left + width, top + height), (0, 255, 0), 2)
cv2.putText(img, label, (left, top - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 输出检测框的左上角和右下角坐标
print("检测到 {},左上角坐标:({},{}),右下角坐标:({}, {})".format(label, left, top, left + width, top + height))
# 显示检测结果
cv2.imshow("Screen", img)
cv2.waitKey(1)
end_time = time.time()
fps = 1 / (end_time - start_time)
print("FPS: {:.2f}".format(fps))
```
该代码会在控制台输出检测框的左上角和右下角坐标,方便后续的操作。同时,该代码可能会占用较高的 CPU 和内存资源,需要注意设备性能。
相关推荐
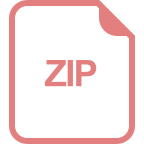
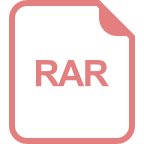
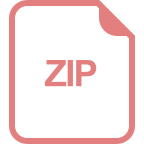














