利用shape类其派生类Rectangle实现矩形绘制,实现鼠标左键按下即在点击处绘制尺寸固定的矩形
时间: 2024-12-23 12:16:53 浏览: 5
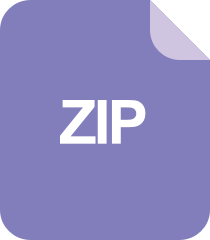
class_shape.zip_class Shape_shape类继承
在图形用户界面编程中,可以使用形状类如Shape,并让Rectangle作为它的派生类来实现矩形的绘制功能。以下是基本步骤:
1. **创建Shape类**:首先定义一个基础Shape类,包含一些通用属性(例如颜色、位置等),以及绘制的方法。
```java
public abstract class Shape {
protected Color color;
protected Point position;
public Shape(Color color, Point position) {
this.color = color;
this.position = position;
}
// 抽象方法,用于绘制形状
public abstract void draw();
}
```
2. **Rectangle类**:派生自Shape,添加特定于矩形的属性(比如宽度和高度),并重写draw()方法,实现在指定位置绘制矩形。
```java
public class Rectangle extends Shape {
private int width;
private int height;
public Rectangle(Color color, Point position, int width, int height) {
super(color, position);
this.width = width;
this.height = height;
}
@Override
public void draw() {
// 实现绘制矩形的逻辑,如使用Graphics API
System.out.println("Drawing a rectangle with size " + width + "x" + height + " at position (" + position.getX() + ", " + position.getY() + ")");
}
}
```
3. **鼠标事件处理**:在GUI组件上监听鼠标的左键按下事件,创建一个新的Rectangle实例并在点击的位置绘制。
```java
MouseListener listener = new MouseAdapter() {
@Override
public void mousePressed(MouseEvent e) {
int x = e.getX();
int y = e.getY();
Rectangle rect = new Rectangle(Color.RED, new Point(x, y), 50, 50); // 假设默认大小为50x50
rect.draw(); // 绘制矩形
}
};
yourComponent.addMouseListener(listener); // 将监听器添加到需要绘制的组件上
```
当你在窗口上按住左键并移动鼠标时,将会在当前位置绘制尺寸为50x50的新矩形。当然,实际应用中会涉及到更复杂的绘图库API,如JavaFX或Swing。
阅读全文
相关推荐
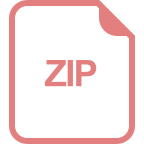
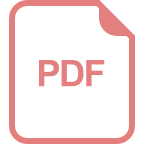















