在c语言中定义顺序表的存储结构及基本操作lnitList,ListLength,GetElem,ListInsert
时间: 2024-10-09 17:05:22 浏览: 59
在C语言中,定义顺序表(也称为数组列表)通常会使用数组作为存储结构,每个元素在内存上连续存放。以下是简单的顺序表及其基本操作的定义:
1. **存储结构**:
```c
typedef struct {
int capacity; // 存储容量
int size; // 当前元素个数
int* elements; // 指向元素的指针数组
} List;
```
这里的`capacity`表示预设的最大元素数量,`size`记录当前实际元素的数量,`elements`是一个指向整型数组的指针。
2. **初始化函数 (initList)**:
```c
void initList(List* list) {
list->capacity = 10; // 初始化容量为10
list->size = 0; // 初始时列表为空
list->elements = malloc(list->capacity * sizeof(int)); // 分配内存空间
}
```
这个函数分配了足够的内存,并设置初始状态。
3. **获取元素长度 (ListLength)**:
```c
int ListLength(const List* list) {
return list->size;
}
```
这个函数返回列表中的元素数量。
4. **获取元素 (GetElem)**:
```c
int GetElem(const List* list, int index) {
if (index < 0 || index >= list->size) {
printf("Index out of range.\n");
return -1; // 或者抛出错误
}
return list->elements[index];
}
```
这个函数用于根据索引访问并返回指定位置的元素,如果索引无效则返回错误值。
5. **插入元素 (ListInsert)**:
```c
void ListInsert(List* list, int index, int value) {
if (index < 0 || index > list->size) {
printf("Invalid insert position.\n");
return;
}
// 如果需要扩大容量,先检查是否已满
if (list->size == list->capacity) {
int newCapacity = list->capacity + (list->capacity / 2); // 动态扩容
int* newArray = realloc(list->elements, newCapacity * sizeof(int));
if (!newArray) {
perror("Memory allocation failed.");
return;
}
list->elements = newArray;
list->capacity = newCapacity;
}
memmove(&list->elements[index+1], &list->elements[index], (list->size - index) * sizeof(int)); // 移动元素
list->elements[index] = value; // 插入新值
list->size++;
}
```
这个函数在指定位置插入新元素,并处理可能出现的动态扩容情况。
阅读全文
相关推荐
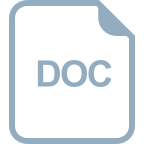
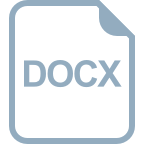
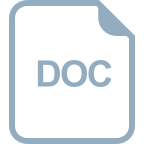


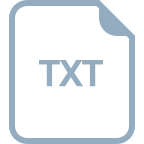
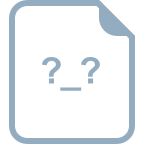
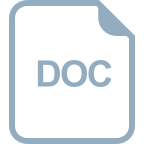
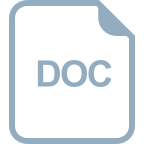
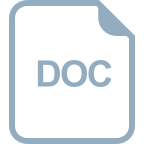
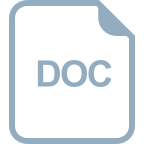
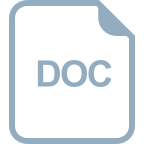
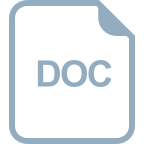






