avl实现学生管理系统c++代码
时间: 2023-12-31 10:02:19 浏览: 32
AVL树是一种自平衡的二叉搜索树,可以用于实现学生管理系统的C代码。以下是一个简单的实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 学生结构体
typedef struct student {
int id;
char name[20];
struct student* left;
struct student* right;
int height;
} Student;
// 获取树的高度
int getHeight(Student* node) {
if (node == NULL) {
return 0;
}
return node->height;
}
// 计算平衡因子
int getBalance(Student* node) {
if (node == NULL) {
return 0;
}
return getHeight(node->left) - getHeight(node->right);
}
// 创建一个新的学生节点
Student* createStudent(int id, char* name) {
Student* node = (Student*)malloc(sizeof(Student));
node->id = id;
strcpy(node->name, name);
node->left = NULL;
node->right = NULL;
node->height = 1;
return node;
}
// 向AVL树插入一个节点
Student* insertStudent(Student* root, int id, char* name) {
if (root == NULL) {
return createStudent(id, name);
}
if (id < root->id) {
root->left = insertStudent(root->left, id, name);
} else if (id > root->id) {
root->right = insertStudent(root->right, id, name);
} else {
printf("节点已存在,无法插入重复的ID\n");
return root;
}
// 更新节点的高度
root->height = 1 + fmax(getHeight(root->left), getHeight(root->right));
// 检查平衡并进行相应的旋转操作
int balance = getBalance(root);
if (balance > 1 && id < root->left->id) {
// 左左情况下的旋转
return rotateRight(root);
}
if (balance < -1 && id > root->right->id) {
// 右右情况下的旋转
return rotateLeft(root);
}
if (balance > 1 && id > root->left->id) {
// 左右情况下的旋转
root->left = rotateLeft(root->left);
return rotateRight(root);
}
if (balance < -1 && id < root->right->id) {
// 右左情况下的旋转
root->right = rotateRight(root->right);
return rotateLeft(root);
}
return root;
}
// 右旋转
Student* rotateRight(Student* y) {
Student* x = y->left;
Student* T2 = x->right;
// 执行旋转
x->right = y;
y->left = T2;
// 更新节点高度
y->height = 1 + fmax(getHeight(y->left), getHeight(y->right));
x->height = 1 + fmax(getHeight(x->left), getHeight(x->right));
return x;
}
// 左旋转
Student* rotateLeft(Student* x) {
Student* y = x->right;
Student* T2 = y->left;
// 执行旋转
y->left = x;
x->right = T2;
// 更新节点高度
x->height = 1 + fmax(getHeight(x->left), getHeight(x->right));
y->height = 1 + fmax(getHeight(y->left), getHeight(y->right));
return y;
}
// 中序遍历打印学生信息
void inorderTraversal(Student* root) {
if (root != NULL) {
inorderTraversal(root->left);
printf("ID: %d, 姓名: %s\n", root->id, root->name);
inorderTraversal(root->right);
}
}
int main() {
Student* root = NULL;
// 插入学生节点
root = insertStudent(root, 1001, "小明");
root = insertStudent(root, 1002, "小红");
root = insertStudent(root, 1003, "小刚");
root = insertStudent(root, 1004, "小李");
// 打印学生信息
inorderTraversal(root);
return 0;
}
```
这段代码实现了向AVL树中插入学生节点,并通过中序遍历打印出学生信息。在插入节点时,会自动进行平衡操作以保证树的平衡性。
相关推荐
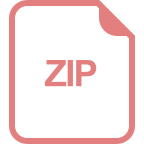
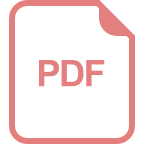














