编写一个学生和教师数据输入和显示程序,学生数据有编号、姓名、班号和成绩,教师数据有编号、姓名、职称和部门。要求将编号、姓名输入和显示设计成一个类person,并作为学生数据操作类student和教师数据操作类teacher的基类。
时间: 2024-06-10 08:06:34 浏览: 112
好的,这个问题属于计算机科学的范畴,可以回答。实现这个程序的关键在于定义一个person类,然后让student和teacher类都继承自person类。person类中需要包括编号和姓名两个属性,并且需要实现输入和显示的方法。student和teacher类需要在person类的基础上添加额外的属性,并且实现相关的操作方法。具体的实现细节需要根据编程语言来确定。
相关问题
编写一个学生和教师数据输入和显示程序,学生数据有编号、姓名、班号和成绩教师数据有编号、姓名、职称和部门。要求将编号、姓名的输入和显示设计成
函数,班号、成绩、职称、部门可以直接在主函数中输入和显示。
以下是示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
struct Student {
int id;
string name;
int classNum;
double score;
};
struct Teacher {
int id;
string name;
string title;
string department;
};
void inputIdAndName(int& id, string& name) {
cout << "请输入编号:";
cin >> id;
cout << "请输入姓名:";
cin >> name;
}
void displayIdAndName(int id, string name) {
cout << "编号:" << id << endl;
cout << "姓名:" << name << endl;
}
int main() {
Student stu;
Teacher tea;
// 输入学生数据
cout << "请输入学生数据:" << endl;
inputIdAndName(stu.id, stu.name);
cout << "请输入班号:";
cin >> stu.classNum;
cout << "请输入成绩:";
cin >> stu.score;
// 显示学生数据
cout << "学生数据:" << endl;
displayIdAndName(stu.id, stu.name);
cout << "班号:" << stu.classNum << endl;
cout << "成绩:" << stu.score << endl;
// 输入教师数据
cout << "请输入教师数据:" << endl;
inputIdAndName(tea.id, tea.name);
cout << "请输入职称:";
cin >> tea.title;
cout << "请输入部门:";
cin >> tea.department;
// 显示教师数据
cout << "教师数据:" << endl;
displayIdAndName(tea.id, tea.name);
cout << "职称:" << tea.title << endl;
cout << "部门:" << tea.department << endl;
return 0;
}
```
编写一个学生和教师数据输入和显示程序,学生数据有编号、姓名、班号和成绩,教师数据有编号、姓名、职称和部门。要求将编号、姓名输入和显示设计成一个类person,并作为学生数据操作类student和教师数
为了编写一个学生和教师数据输入和显示的程序,我们可以首先创建一个名为`Person`的通用类,用于存储`编号`、`姓名`等基本信息。然后,基于这个类分别创建`Student`和`Teacher`两个子类,每个子类会添加特定的数据字段如`班号`、`成绩` 和 `职称`、`部门`。这里是一个简单的示例:
```python
class Person:
def __init__(self, id, name):
self.id = id
self.name = name
def input_data(self):
print("请输入{}的信息:".format(self.__class__.__name__))
self.id = int(input("编号: "))
self.name = input("姓名: ")
def display_data(self):
print("\n{}信息:\nID: {}\n姓名: {}".format(self.__class__.__name__, self.id, self.name))
class Student(Person):
def __init__(self, id, name, class_number, score):
super().__init__(id, name)
self.class_number = class_number
self.score = score
def input_data(self):
super().input_data()
self.class_number = int(input("班号: "))
self.score = float(input("成绩: "))
def display_data(self):
super().display_data()
print("班号: {}\n成绩: {}".format(self.class_number, self.score))
class Teacher(Person):
def __init__(self, id, name, title, department):
super().__init__(id, name)
self.title = title
self.department = department
def input_data(self):
super().input_data()
self.title = input("职称: ")
self.department = input("部门: ")
def display_data(self):
super().display_data()
print("职称: {}\n部门: {}".format(self.title, self.department))
# 使用示例
student = Student(0, "张三", 1, 85)
teacher = Teacher(1, "李四", "教授", "计算机科学系")
student.input_data()
student.display_data()
teacher.input_data()
teacher.display_data()
```
在这个例子中,`Person` 类负责基本的输入和显示功能,而`Student`和`Teacher`类则扩展了这些功能并添加了各自的特有属性。
阅读全文
相关推荐
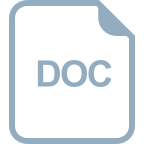
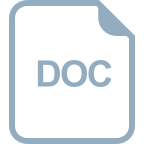
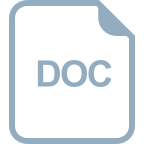










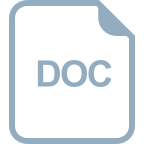
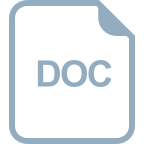
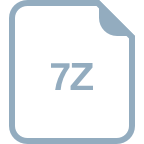
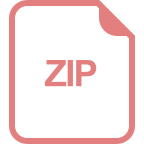