生命游戏C++代码
时间: 2023-07-10 22:14:26 浏览: 65
以下是一个简单的生命游戏C++代码示例,用于演示该游戏的基本规则和逻辑:
```cpp
#include <iostream>
#include <cstdlib>
#include <time.h>
using namespace std;
const int ROWS = 20;
const int COLS = 20;
// 定义棋盘状态,0表示死亡,1表示存活
int board[ROWS][COLS];
// 初始化棋盘
void initBoard() {
srand(time(NULL));
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
board[i][j] = rand() % 2;
}
}
}
// 打印棋盘状态
void printBoard() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
cout << board[i][j] << " ";
}
cout << endl;
}
cout << endl;
}
// 统计周围存活的细胞数
int countLiveCells(int row, int col) {
int count = 0;
for (int i = row-1; i <= row+1; i++) {
for (int j = col-1; j <= col+1; j++) {
if (i >= 0 && i < ROWS && j >= 0 && j < COLS && !(i == row && j == col)) {
count += board[i][j];
}
}
}
return count;
}
// 更新棋盘状态
void updateBoard() {
int newBoard[ROWS][COLS];
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
int liveCells = countLiveCells(i, j);
if (board[i][j] == 1) {
if (liveCells < 2 || liveCells > 3) {
newBoard[i][j] = 0;
} else {
newBoard[i][j] = 1;
}
} else {
if (liveCells == 3) {
newBoard[i][j] = 1;
} else {
newBoard[i][j] = 0;
}
}
}
}
memcpy(board, newBoard, sizeof(board));
}
int main() {
initBoard();
for (int i = 0; i < 10; i++) {
printBoard();
updateBoard();
}
return 0;
}
```
该代码使用了一个二维数组来表示棋盘状态,其中0表示死亡,1表示存活。在每一轮更新中,我们统计每个细胞周围存活的细胞数,根据规则来更新其状态。最后,我们通过多次循环来模拟生命游戏的演化过程。
相关推荐
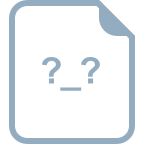













