生命游戏C++如何在终端改变细胞状态代码
时间: 2024-03-18 11:39:15 浏览: 17
生命游戏是一种基于细胞自动机的模拟游戏,其中每个细胞的状态会随着时间的推移而改变。在 C++ 中实现生命游戏,可以使用二维数组来表示细胞的状态,然后通过循环遍历数组,根据细胞周围的状态来计算细胞下一时刻的状态。
要在终端改变细胞的状态,可以使用标准输入输出流。具体操作可以参考下面的代码示例:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
// 定义细胞状态
const int ALIVE = 1;
const int DEAD = 0;
// 定义细胞矩阵大小
const int ROWS = 10;
const int COLS = 10;
// 定义细胞矩阵
int cells[ROWS][COLS];
// 初始化细胞状态
void initCells() {
srand(time(NULL));
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
cells[i][j] = rand() % 2;
}
}
}
// 显示细胞矩阵
void displayCells() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (cells[i][j] == ALIVE) {
cout << "* ";
} else {
cout << ". ";
}
}
cout << endl;
}
}
// 计算细胞下一时刻的状态
void calculateNextState() {
int nextCells[ROWS][COLS];
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
int neighbors = 0;
for (int m = -1; m <= 1; m++) {
for (int n = -1; n <= 1; n++) {
if (m == 0 && n == 0) {
continue;
}
int row = i + m;
int col = j + n;
if (row < 0 || row >= ROWS || col < 0 || col >= COLS) {
continue;
}
if (cells[row][col] == ALIVE) {
neighbors++;
}
}
}
if (cells[i][j] == ALIVE) {
if (neighbors < 2 || neighbors > 3) {
nextCells[i][j] = DEAD;
} else {
nextCells[i][j] = ALIVE;
}
} else {
if (neighbors == 3) {
nextCells[i][j] = ALIVE;
} else {
nextCells[i][j] = DEAD;
}
}
}
}
// 更新细胞状态
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
cells[i][j] = nextCells[i][j];
}
}
}
int main() {
initCells();
displayCells();
while (true) {
cout << "请输入要改变状态的细胞坐标(如:2 3):";
int x, y;
cin >> x >> y;
if (x < 0 || x >= ROWS || y < 0 || y >= COLS) {
cout << "坐标不合法,请重新输入!" << endl;
continue;
}
if (cells[x][y] == ALIVE) {
cells[x][y] = DEAD;
} else {
cells[x][y] = ALIVE;
}
displayCells();
calculateNextState();
displayCells();
}
return 0;
}
```
在这个示例中,我们使用二维数组 `cells` 来表示细胞状态,通过 `initCells` 函数初始化细胞状态,通过 `displayCells` 函数显示细胞矩阵。在 `calculateNextState` 函数中,我们计算细胞下一时刻的状态,然后更新细胞状态。在主函数中,我们通过标准输入输出流来获取用户输入的细胞坐标,然后改变细胞的状态,并显示细胞矩阵和计算后的下一时刻细胞矩阵。
相关推荐
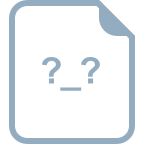
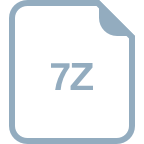














