生命游戏C++如何在终端改变细胞状态代码 并用类和对象
时间: 2024-03-18 07:39:23 浏览: 16
可以使用类和对象来实现生命游戏,其中类表示细胞自动机,对象表示细胞矩阵。具体操作可以参考下面的代码示例:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
// 定义细胞状态
const int ALIVE = 1;
const int DEAD = 0;
// 定义细胞矩阵大小
const int ROWS = 10;
const int COLS = 10;
// 定义细胞自动机类
class CellAutoMaton {
public:
CellAutoMaton() {
// 初始化细胞状态
srand(time(NULL));
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
cells[i][j] = rand() % 2;
}
}
}
// 显示细胞矩阵
void displayCells() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (cells[i][j] == ALIVE) {
cout << "* ";
} else {
cout << ". ";
}
}
cout << endl;
}
}
// 改变细胞状态
void changeCellState(int x, int y) {
if (x < 0 || x >= ROWS || y < 0 || y >= COLS) {
cout << "坐标不合法,请重新输入!" << endl;
return;
}
if (cells[x][y] == ALIVE) {
cells[x][y] = DEAD;
} else {
cells[x][y] = ALIVE;
}
}
// 计算细胞下一时刻的状态
void calculateNextState() {
int nextCells[ROWS][COLS];
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
int neighbors = 0;
for (int m = -1; m <= 1; m++) {
for (int n = -1; n <= 1; n++) {
if (m == 0 && n == 0) {
continue;
}
int row = i + m;
int col = j + n;
if (row < 0 || row >= ROWS || col < 0 || col >= COLS) {
continue;
}
if (cells[row][col] == ALIVE) {
neighbors++;
}
}
}
if (cells[i][j] == ALIVE) {
if (neighbors < 2 || neighbors > 3) {
nextCells[i][j] = DEAD;
} else {
nextCells[i][j] = ALIVE;
}
} else {
if (neighbors == 3) {
nextCells[i][j] = ALIVE;
} else {
nextCells[i][j] = DEAD;
}
}
}
}
// 更新细胞状态
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
cells[i][j] = nextCells[i][j];
}
}
}
private:
int cells[ROWS][COLS];
};
int main() {
CellAutoMaton lifeGame;
lifeGame.displayCells();
while (true) {
cout << "请输入要改变状态的细胞坐标(如:2 3):";
int x, y;
cin >> x >> y;
lifeGame.changeCellState(x, y);
lifeGame.displayCells();
lifeGame.calculateNextState();
lifeGame.displayCells();
}
return 0;
}
```
在这个示例中,我们定义了一个 `CellAutoMaton` 类来表示细胞自动机,其中包含了细胞矩阵和相关的操作。在构造函数中,我们初始化细胞状态。在 `displayCells` 函数中,我们显示细胞矩阵。在 `changeCellState` 函数中,我们根据用户输入的细胞坐标来改变细胞状态。在 `calculateNextState` 函数中,我们计算细胞下一时刻的状态,并更新细胞状态。在主函数中,我们创建了一个 `CellAutoMaton` 对象,并通过对象来调用相关操作。
相关推荐
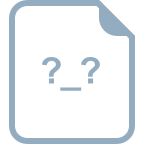
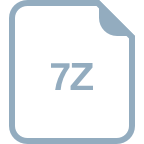














